Interface-methods is a mechanism that the developer platform provides, to enable your app to trigger certain actions on the Freshsales Suite UI.
Using interface methods an app can, on the Freshsales Suite UI,
- Display certain UI elements such as Modals, Confirmation boxes, and Notifications.
- Open specific windows or pages.
Global interfaces
An app can display these interfaces/UI elements on any of the Freshsales Suite pages where the app is deployed. Regardless of where the app is deployed, the app can also open specific windows or pages in the UI.
Display modals
From your project’s root, navigate to the app directory and create a <modal or any-reasonable-name>.html file. In this file, enter the code to render the (front-end of the) Modal.
Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
After app initialization, to display a Modal, use the following method:
Sample app.jsclient.interface.trigger("showModal", { title: "<Modal name>", template: "<path to the modal.html file>" })
showModal method
The method displays a Modal in an IFrame. Events methods and Interface methods are not supported in a Modal - your app cannot react to click, change, or update events that occur inside a Modal and your app cannot trigger actions on the product UI from the Modal.
Installation parameters, Request method, Data method, and Data storage are supported in modals. If your app uses these methods from modals, in the modal.html file (or in any other equivalent file that you have added), ensure to include the following appclient resource.
<script src="{{{appclient}}}"></script>
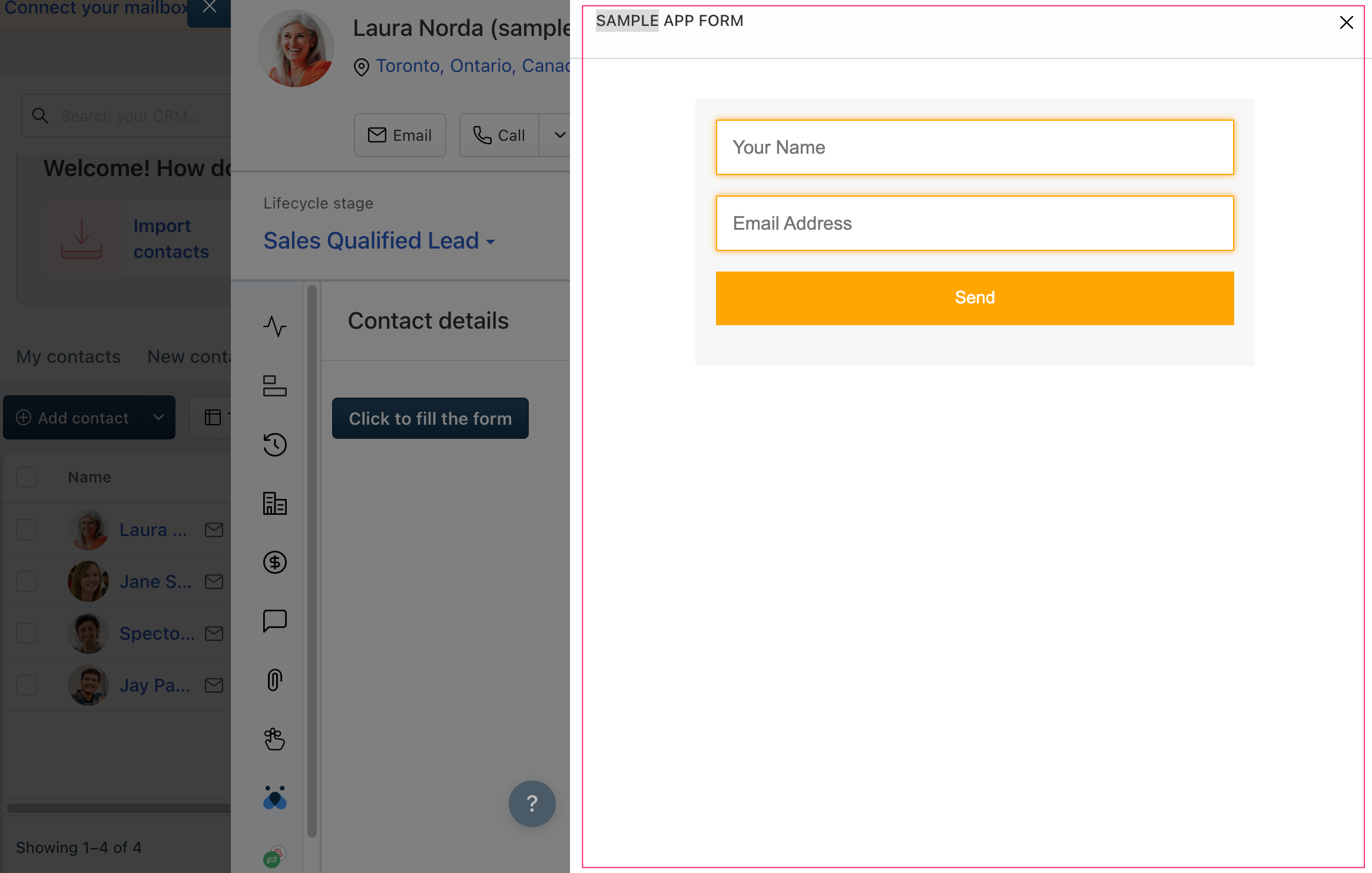
Use the sample code shown on the right pane, to enable your app to display a Modal, as part of the app logic.
You can retrieve the contextual information pertaining to the parent location from where a modal is triggered by using Instance method - Context. You can use the Instance methods - Send and Receive to enable communication between modals and any other app instance.
Display confirmation messages
To enable your app to display standard confirmation-message boxes,
- Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
- After app initialization, to display a confirmation message with default buttons, use the following method:
client.interface.trigger("showConfirm", {
title: "<Confirmation box name>",
message: "<text message to be displayed seeking confirmation>"
})
showConfirm method
The method displays a confirmation message box with a title, a message, and the save and cancel buttons. By default, the message box displays the SAVE and CANCEL buttons. You can customize the button names.
Time-out information:Timeout for Confirmation message box is 10 seconds.
Use the sample code shown on the right pane > Default buttons tab, to enable your app to display a Confirmation message box (with SAVE and CANCEL buttons), as part of the app logic.
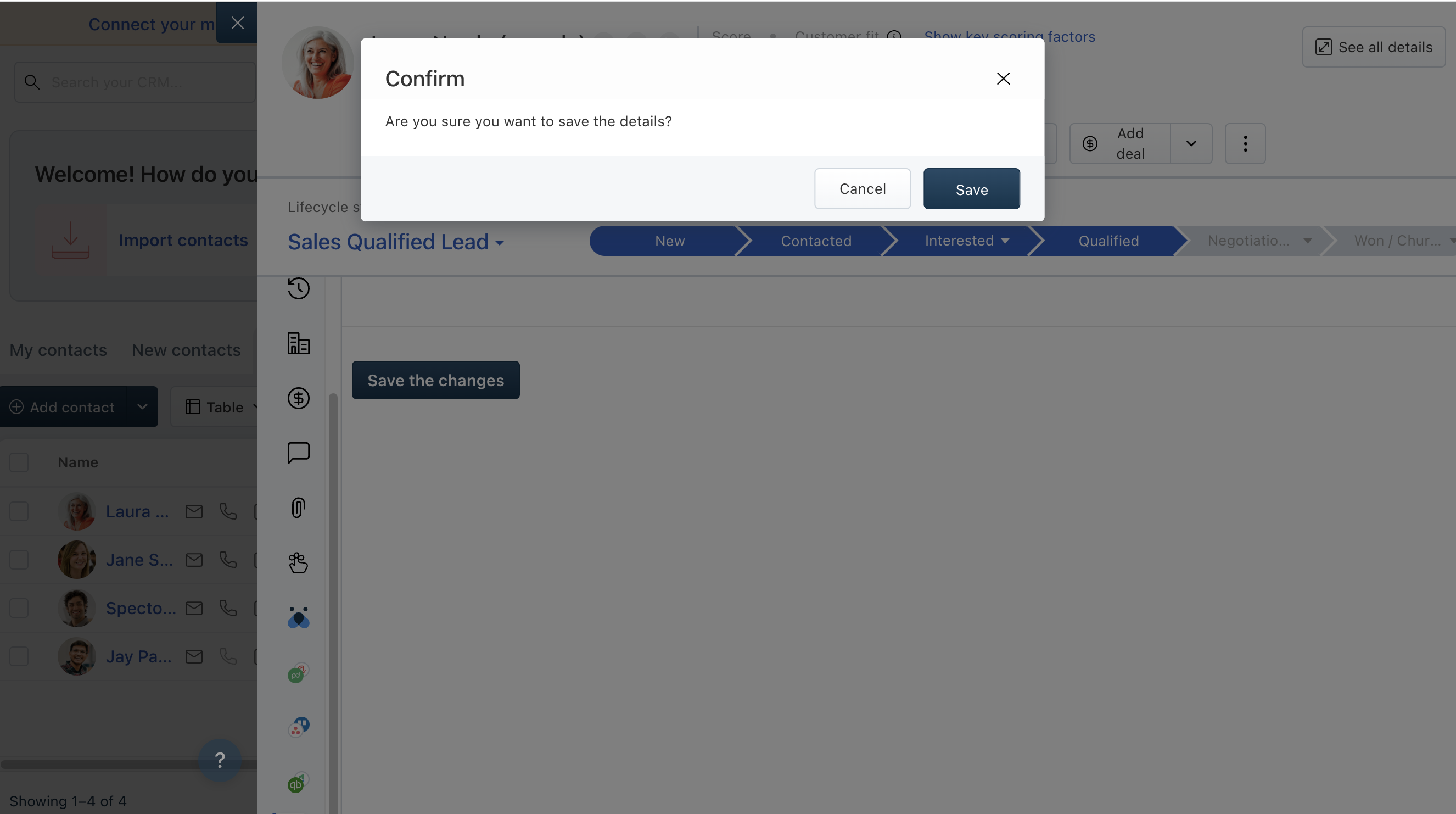
Display notifications
To enable your app to display notifications,
Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
After app initialization, to display a notification, use the following method:
Sample app.jsclient.interface.trigger("showNotify", { type: “<Possible values: info, success, warning, danger>”, title: "<Display name>", message: "<text message to be displayed in the notification box>" } )
showNotify method
The method displays a notification box with a title and notification message (can be an info message, a success message, a cautionary note, or a critical message which, if ignored, can fail the system).
Use the sample code shown on the right pane, to enable your app to display various notification messages, as part of the app logic.
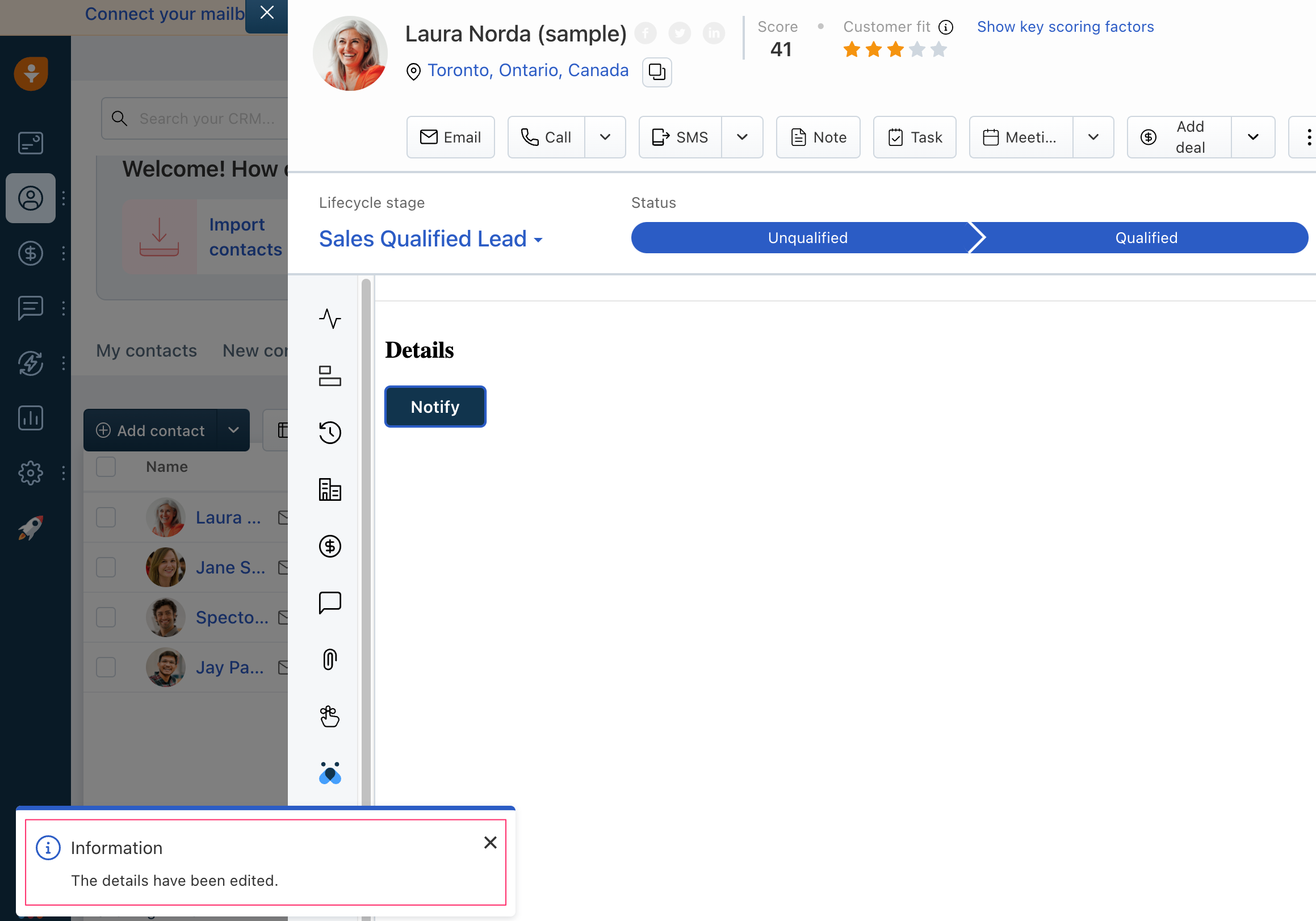
Open ADD CONTACT, ADD ACCOUNT, ADD DEAL, or CALL LOG windows
To enable your app to open the ADD CONTACT, ADD ACCOUNT, ADD DEAL, or CALL LOG windows,
Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (product on which the app is deployed) passes a client reference to the app.
After app initialization, to open specific windows, use the following method:
client.interface.trigger("open", { id: "<window-name>" })
<window-name> is the window to be opened. Possible values: contact, account, deal, calllog
open method
Use the sample code shown on the right pane, to enable your app to open the ADD CONTACT, ADD ACCOUNT, ADD DEAL, or CALL LOG windows.
Open contact, account, or deal details page
To enable your app to open the contact, account, or deal details page,
- Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (product on which the app is deployed) passes a client reference to the app.
- After app initialization, to open specific pages, use the following method:
client.interface.trigger("show", {
id: "<contact, account, or deal>",
value: <id of the contact, account, or deal to be opened>
})
id: "contact" opens the Contact details page of the contact whose contact-id is specified in value.
id: "account" opens the Account details page of the account whose account-id is specified in value.
id: "deal" opens the Deal details page of the deal whose deal-id is specified in value.
show method
The method opens the contact, account, or deal details page of a specific contact, account, or deal. Use the sample code shown on the right pane, to enable your app to open the contact, account, or deal details page.