Interface-methods is a mechanism that the developer platform provides, to enable your app to trigger certain actions on the Freshchat UI.
Using interface methods an app can, on the Freshchat UI,
- Display certain UI elements such as Modals, Dialog boxes, Confirmation boxes, and Notifications.
- Show/Hide certain fields, field values, and elements (such as icons or other relevant information).
- Enable/Disable certain buttons.
- Set or clear the values of certain fields.
Global interfaces
An app can display these interfaces/UI elements on any of the Freshchat pages where the app is deployed.
Display modals or dialog boxes
From your project’s root, navigate to the app directory and create a <modal or dialog or any-reasonable-name>.html file. In this file, enter the code to render the (front-end of the) Modal or Dialog box.
Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
After app initialization, to display a Modal or Dialog box, use the following method:
Sample app.jsclient.interface.trigger("<method-name>", { title: "<Modal name>", template: "<path to the modal.html file>" })
<method-name> is showModal or showDialog
showModal method
The method displays a Modal in an IFrame. Events methods and Interface methods are not supported in a Modal - your app cannot react to click, change, or update events that occur inside a Modal and your app cannot trigger actions on the product UI from the Modal.
Installation parameters, Request method, Data method, and Data storage are supported in modals. If your app uses these methods from modals, in the modal.html file (or in any other equivalent file that you have added), ensure to include the following appclient resource.
<script src="{{{appclient}}}"></script>
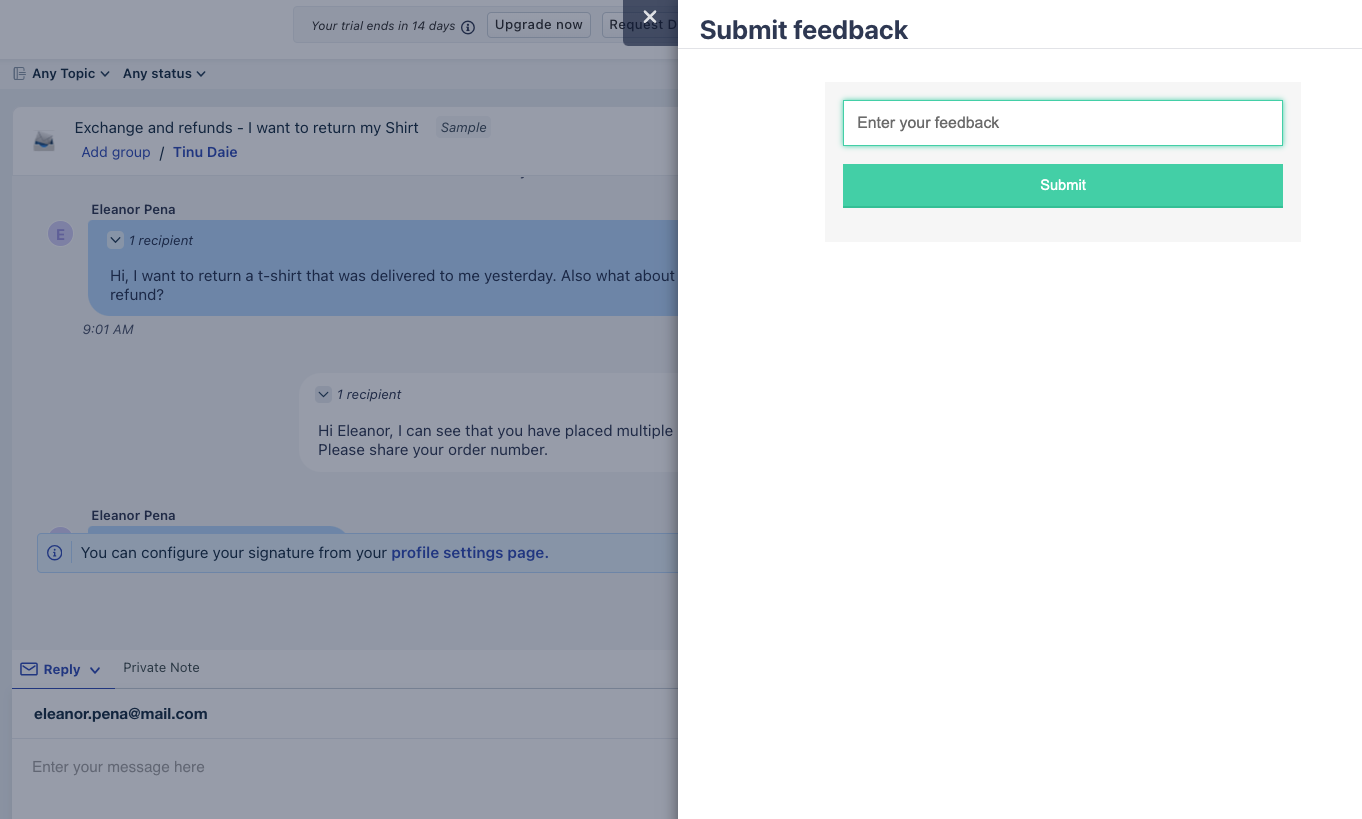
Use the sample code shown on the right pane, to enable your app to display a Modal, as part of the app logic.
You can retrieve the contextual information pertaining to the parent location from where a modal is triggered by using Instance method - Context. You can use the Instance methods - Send and Receive to enable communication between modals and any other app instance.
showDialog method
The method displays a Dialog box in an IFrame. Events methods and Interface methods are not supported in a Dialog box - your app cannot react to click, change, or update front-end events that occur inside a Dialog box and your app cannot trigger actions on the product UI from the Dialog box.
Installation parameters, Request method, Data method, and Data storage are supported in modals. If your app uses these methods from modals, in the dialog.html file (or in any other equivalent file that you have added), ensure to include the following appclient resource.
<script src="{{{appclient}}}"></script>
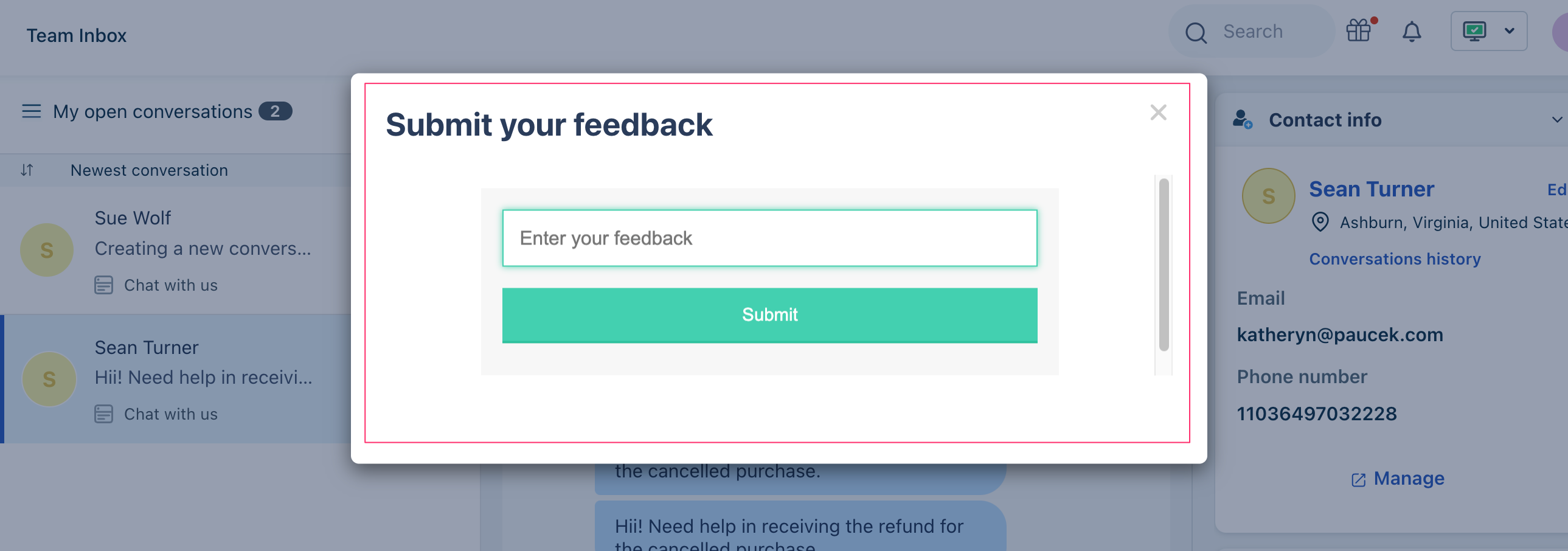
Use the sample code shown on the right pane, to enable your app to display a Dialog box, as part of the app logic.
You can retrieve the contextual information pertaining to the parent location from where a Dialog box is triggered by using Instance method - Context. You can use the Instance methods - Send and Receive to enable communication between modals and any other app instance.
Display confirmation messages
To enable your app to display standard confirmation-message boxes,
- Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
- After app initialization, to display a confirmation message with default buttons, use the following method:
client.interface.trigger("showConfirm", {
title: "<Confirmation box name>",
message: "<text message to be displayed seeking confirmation>"
})
showConfirm method
The method displays a confirmation message box with a title, a message, and the save and cancel buttons. By default, the message box displays the SAVE and CANCEL buttons. You can customize the button names.
Time-out information:Timeout for Confirmation message box is 10 seconds.
Use the sample code shown on the right pane > Default buttons tab, to enable your app to display a Confirmation message box (with SAVE and CANCEL buttons), as part of the app logic.
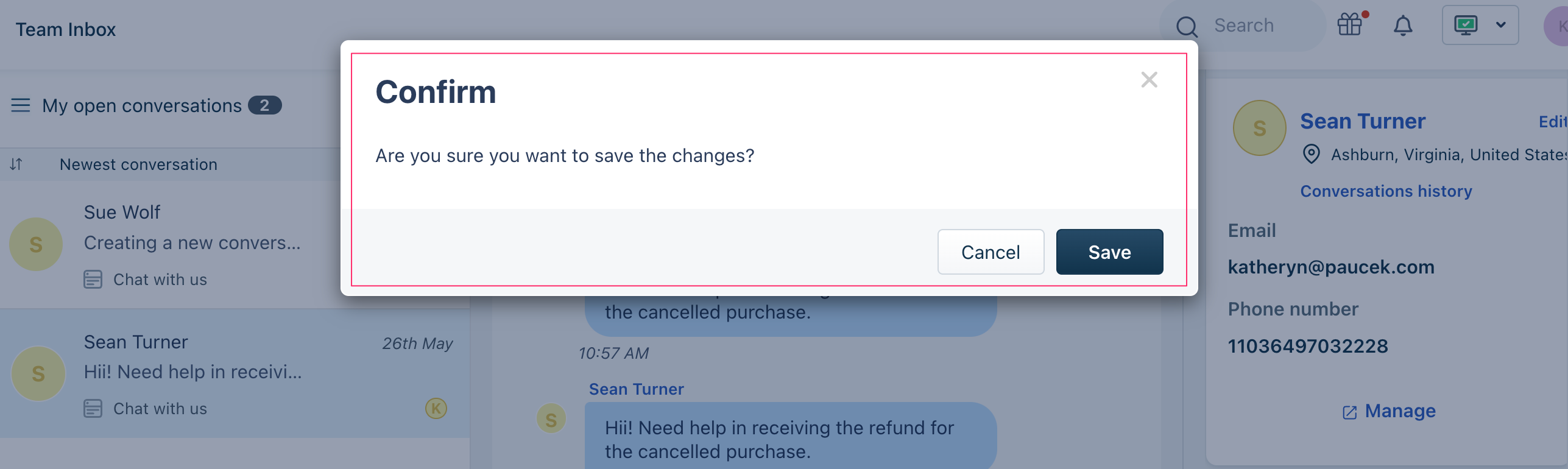
Display notifications
To enable your app to display notifications,
Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
After app initialization, to display a notification, use the following method:
Sample app.jsclient.interface.trigger("showNotify", { type: “<Possible values: info, success, warning, alert, danger>”, message: "<text message to be displayed in the notification box>" } )
showNotify method
The method displays a notification box with a title and notification message (can be which, if ignored, can fail the system).
Use the sample code shown on the right pane, to enable your app to display various notification messages, as part of the app logic.
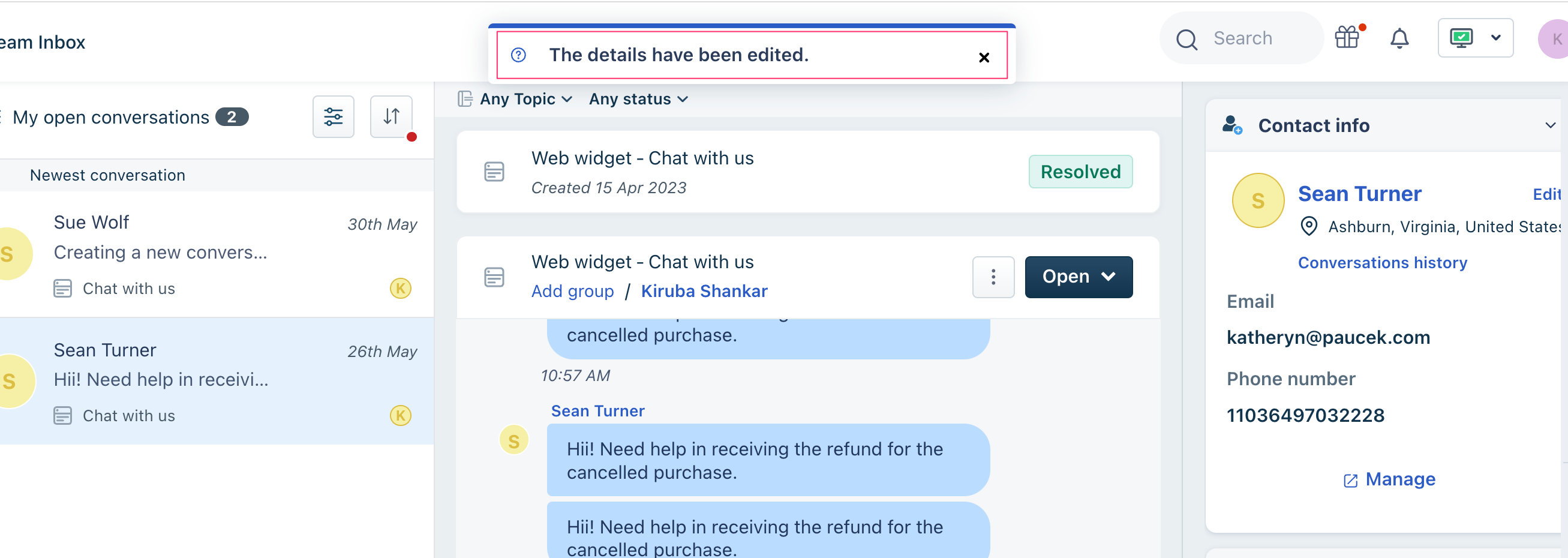
Hide/display or enable/disable UI elements
TTo enable your app to hide an UI element, display a hidden element, disable an element, or enable an element,
- Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
- After app initialization, to a hide/display or enable/disable UI element, use the following method:<method-name> is the action to be performed. Possible values: hide, show, enable, disableapp.js
client.interface.trigger("<method-name>", { id: "<element-name>" })
<element-name> is the element to be hidden or displayed; disabled or enabled.
Set the value of a field
To enable your app to set the value of a UI field,
Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
After app initialization, use the following method:
app.jsclient.interface.trigger("setValue", { id: "<element-name>", value: "<value to be set>" })
<element-name> is the element whose value is to be set.
Clear the value of a field
To enable your app to clear the value of a UI field,
Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
After app initialization, use the following method:
app.jsclient.interface.trigger("clearValue", { id: "<element-name>" })
<element-name> is the element whose value is to be cleared.
UI elements that the app can act on
addAttachment
Use the sample code on the right pane, to hide or display the Attach File icon.
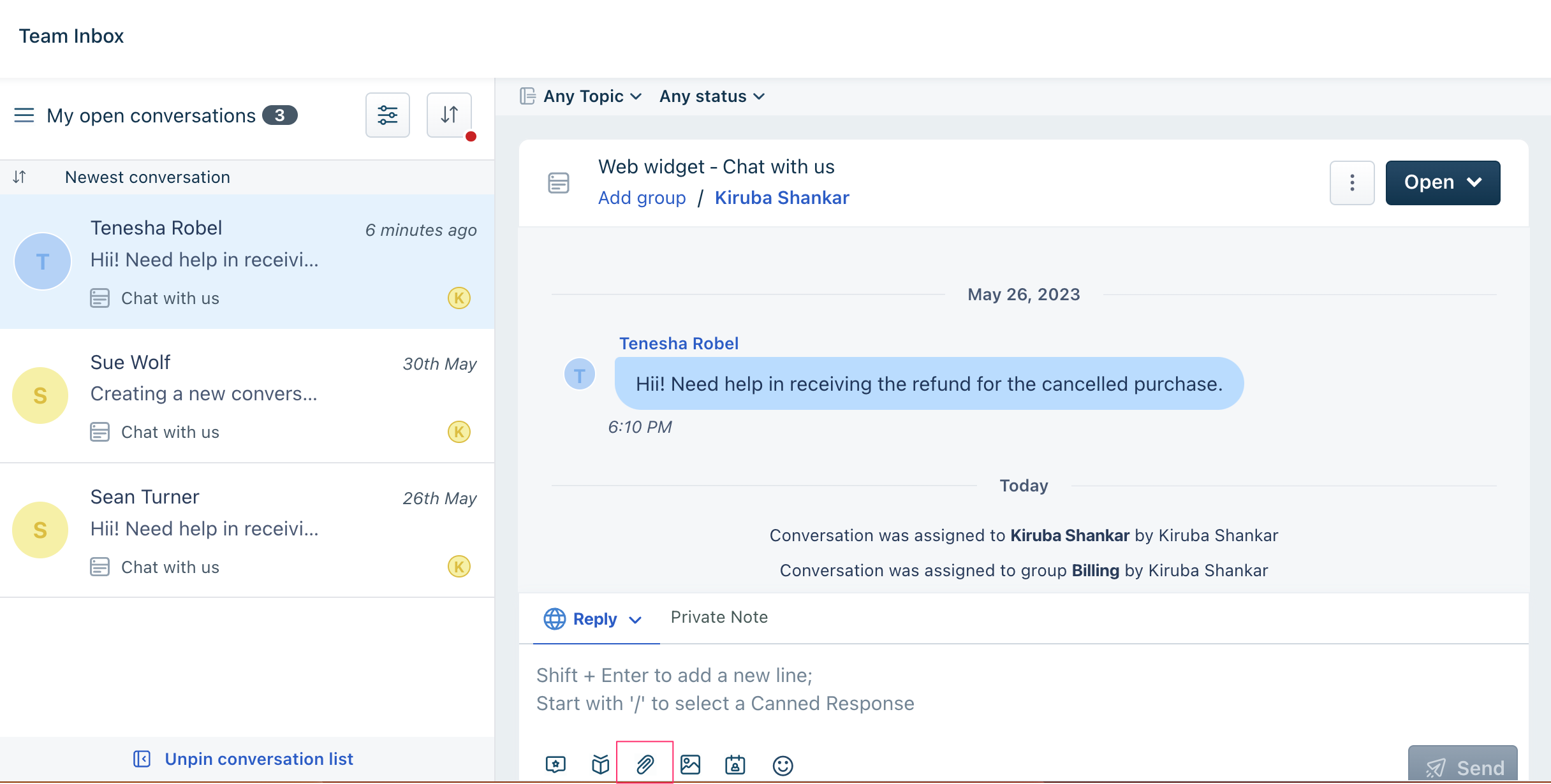
addImage
Use the sample code on the right pane, to hide or display the Add Image icon.
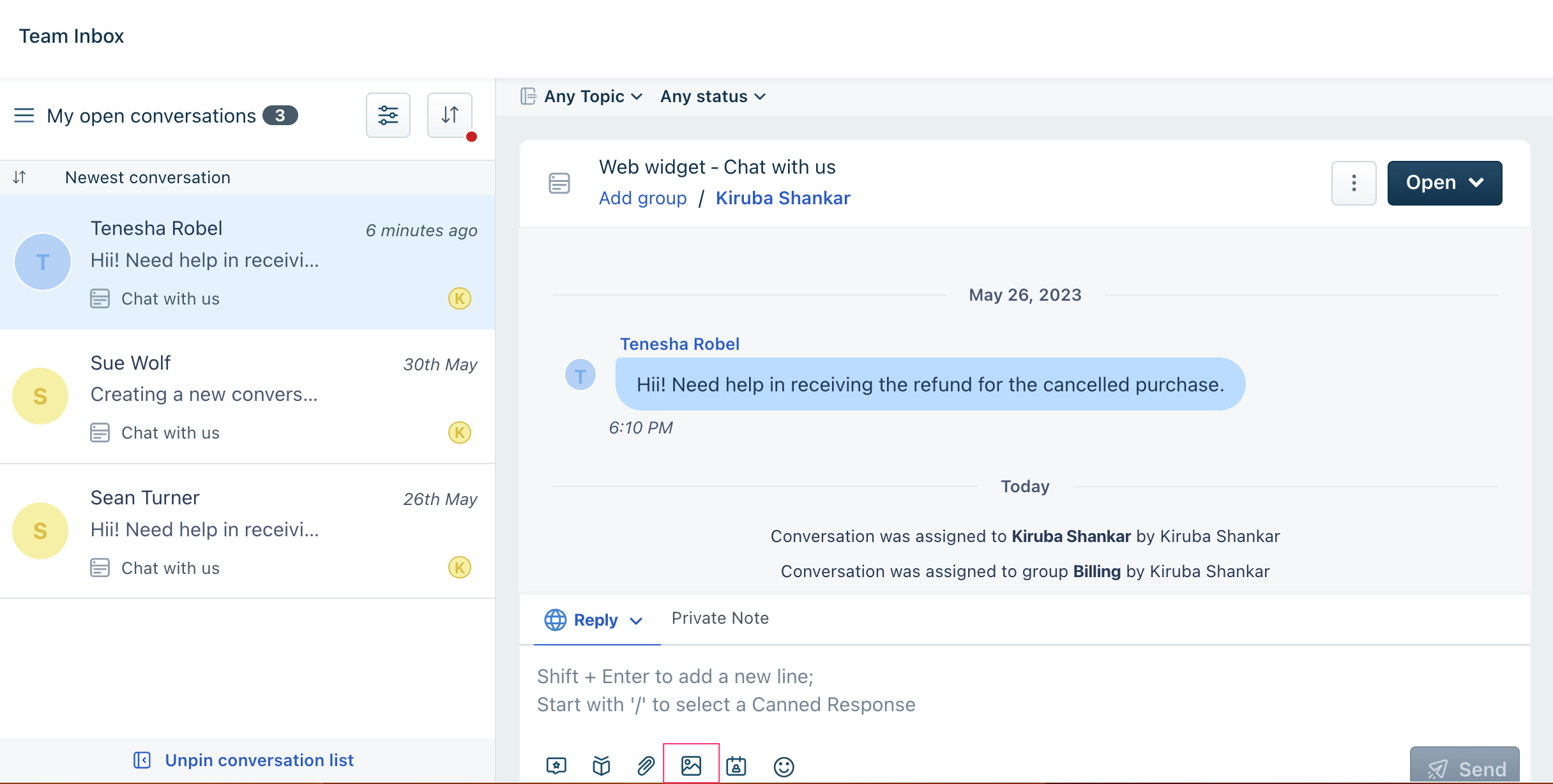
coBrowse
Use the sample code on the right pane, to show or hide the Co-browse icon.
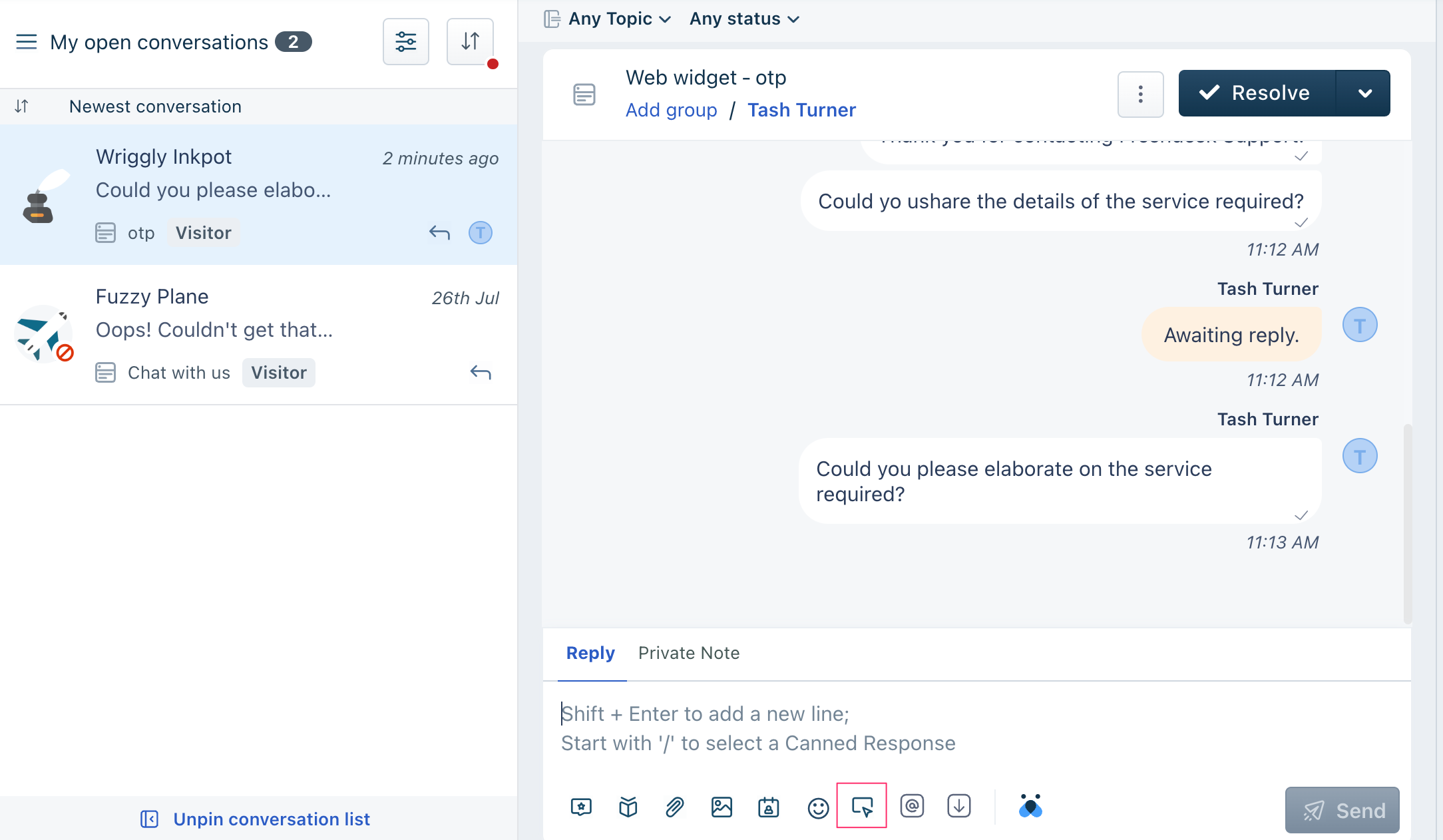
resolveConversation
Use the sample code on the right pane, to disable or enable the Resolve button.
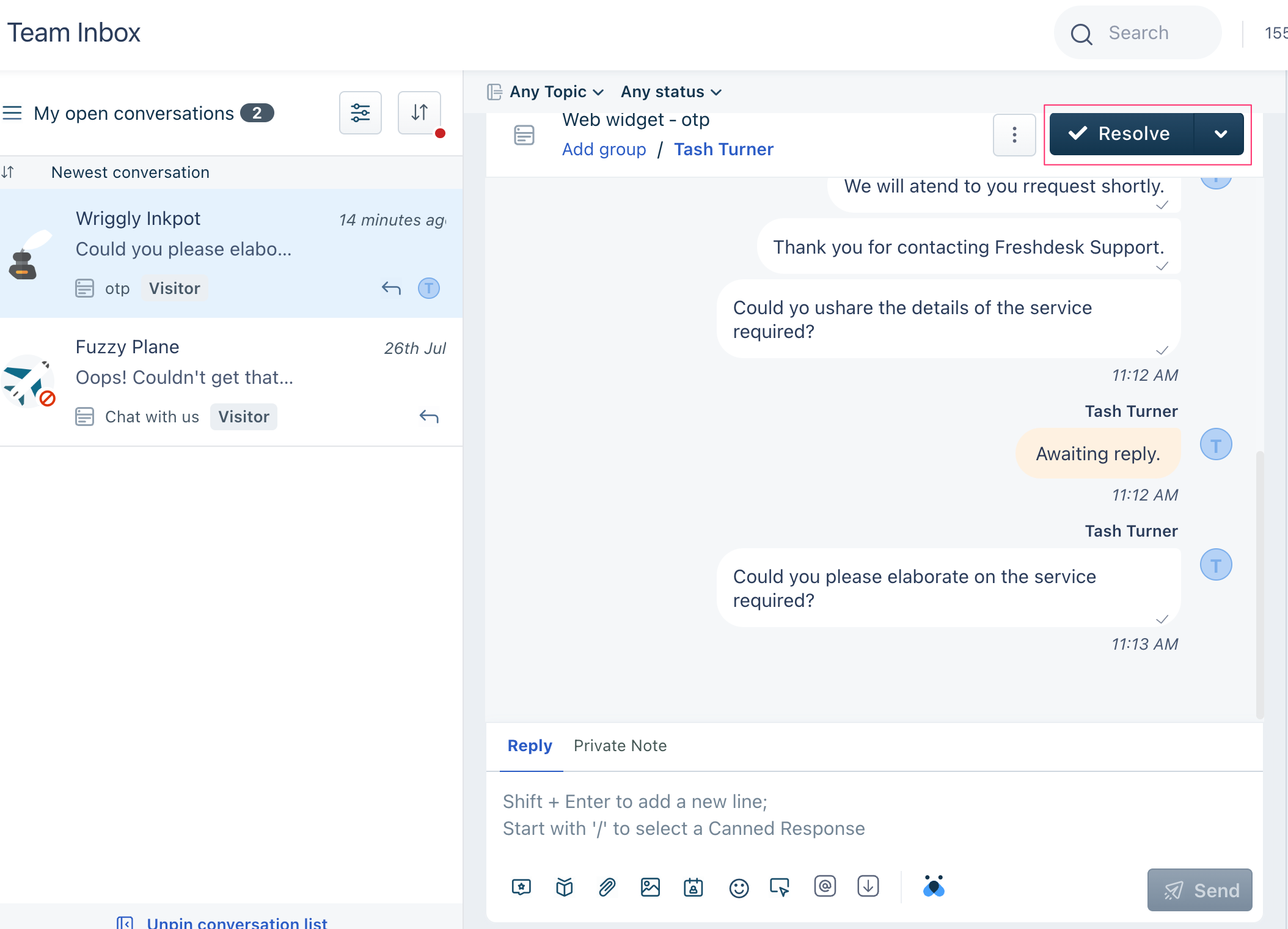
If the app is deployed on a Freshchat account with the <sub-domain>.myfreshworks.com account URL, on the conversation window, there is an Open drop-down button with Resolve as a menu option. When the enable or disable method acts on the resolveConversation element, the Open button is disabled or enabled.
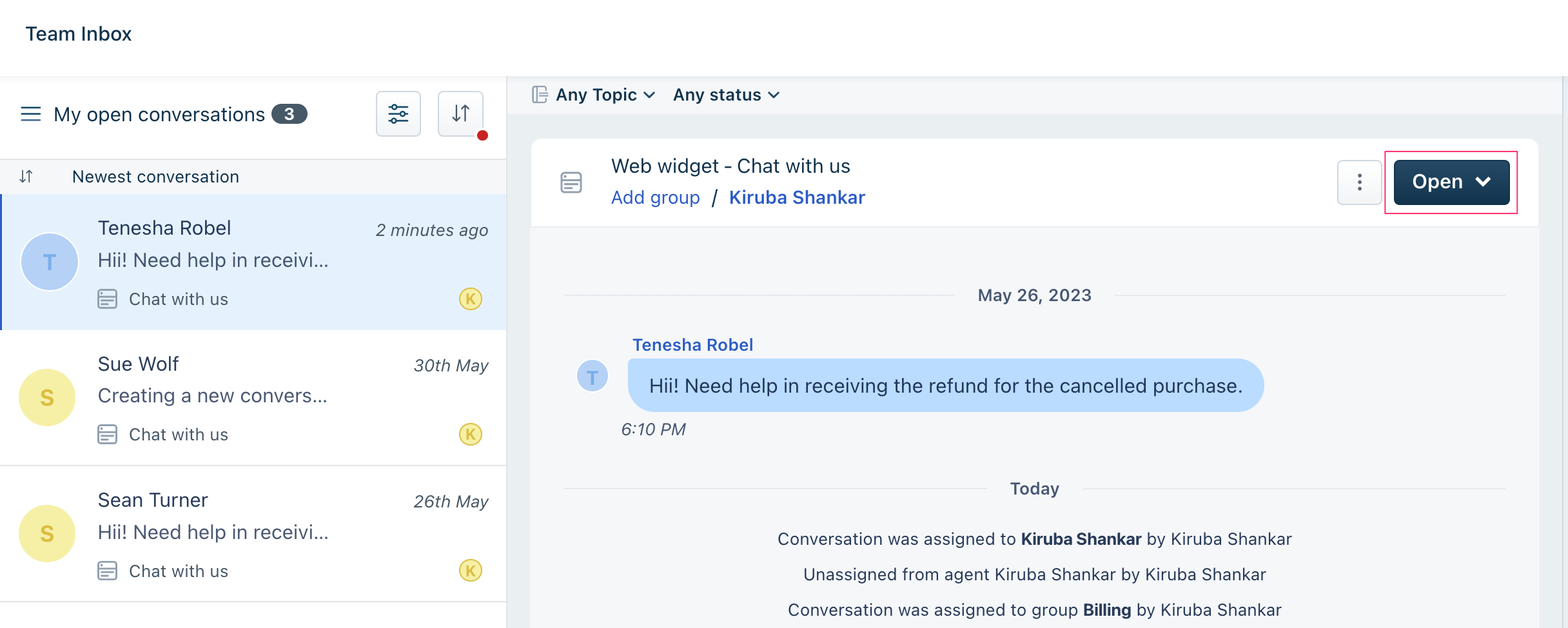
assignGroup
Use the sample code shown on the right pane, to enable or disable the Add group element from the conversation window. If a group is assigned, the window contains the group’s name in place of the Add group element. In that case, the group’s name is disabled or enabled when the disable or enable method is used on the assignGroup element.
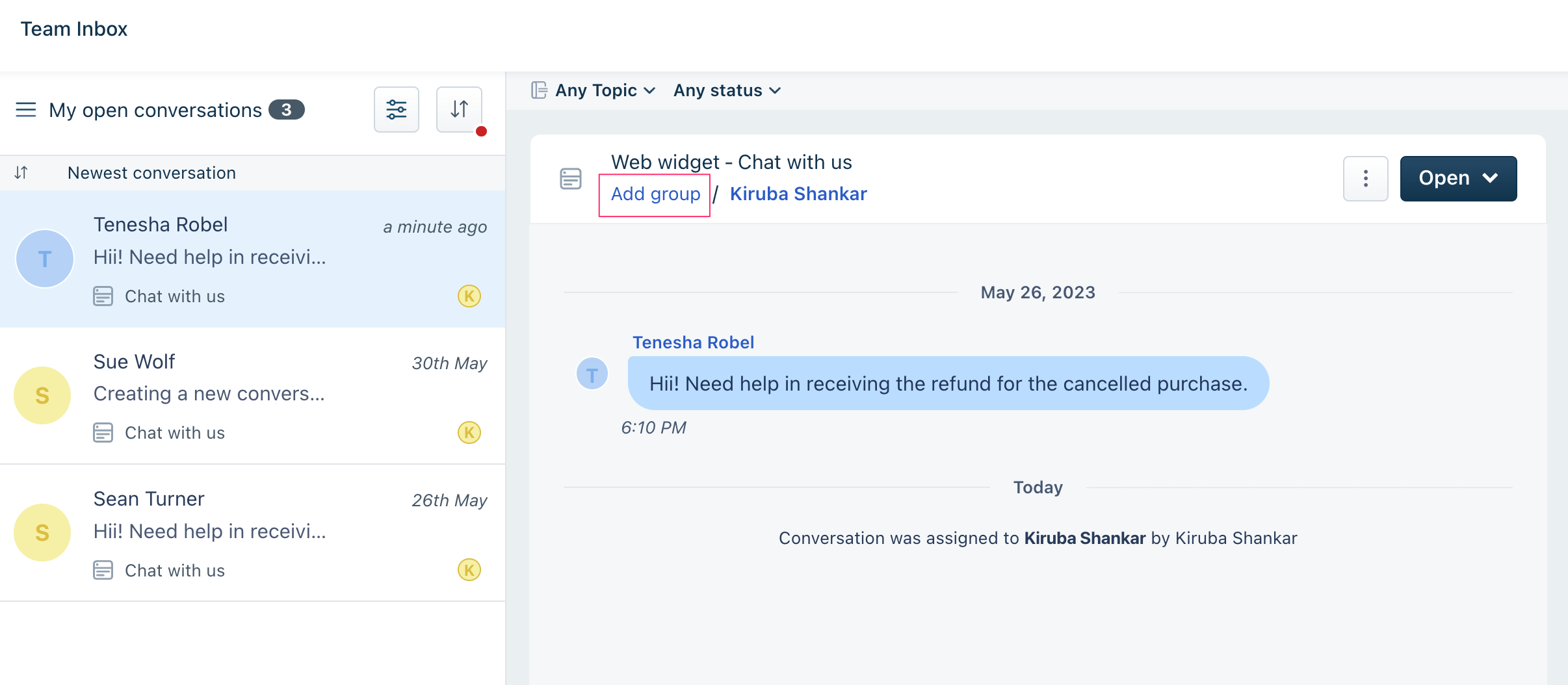
assignAgent
Use the sample code shown on the right pane, to enable or disable the Add agent element from the conversation window. If an agent is assigned, the window contains the agent’s name in place of the Add agent element. In that case, the agent’s name is disabled or enabled when the disable or enable method is used on the assignAgent element.
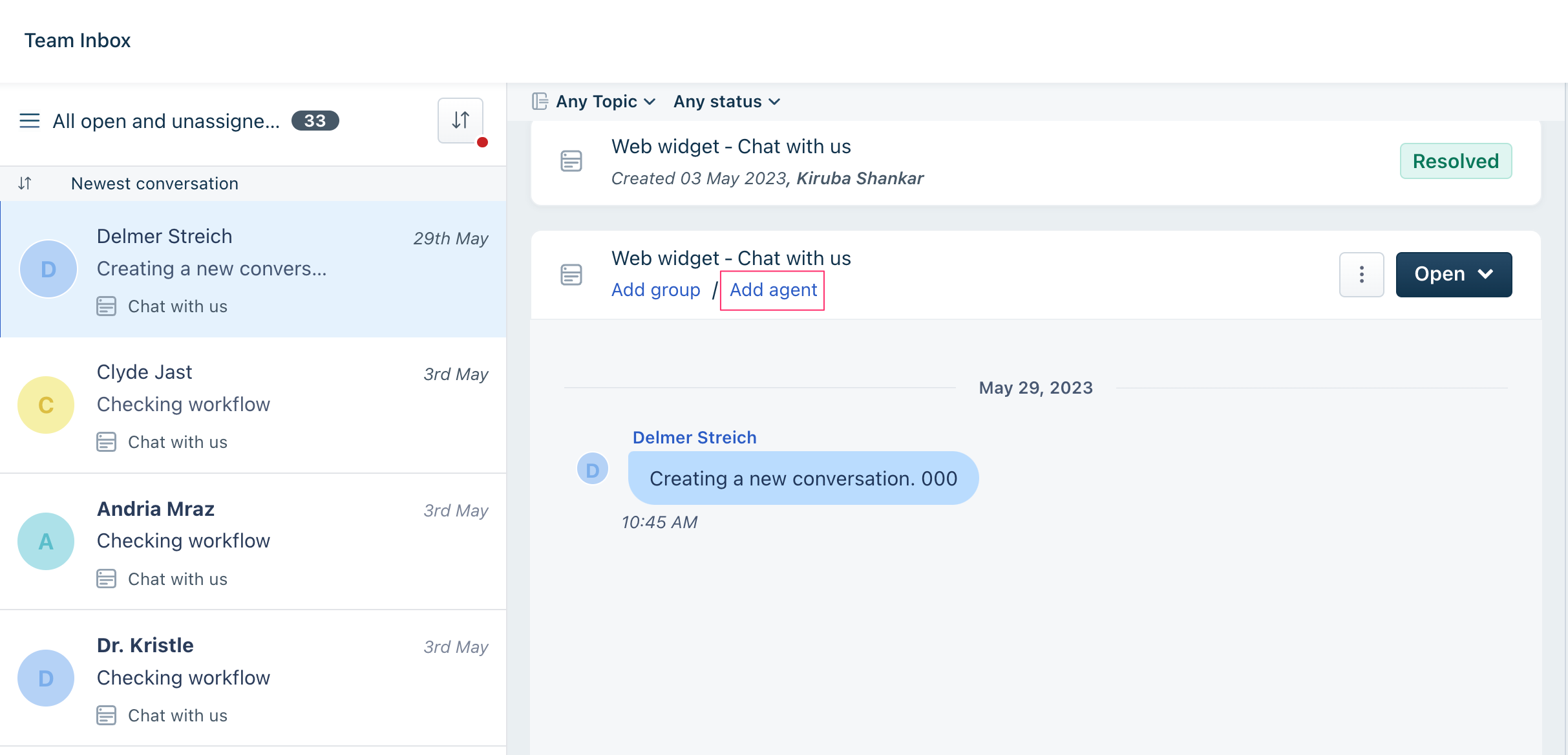
Contact info widget fields
The product UI displays all information pertaining to the contact (user) associated with a conversation in the Contact info widget of the Team Inbox page. The widget is highlighted in the image.
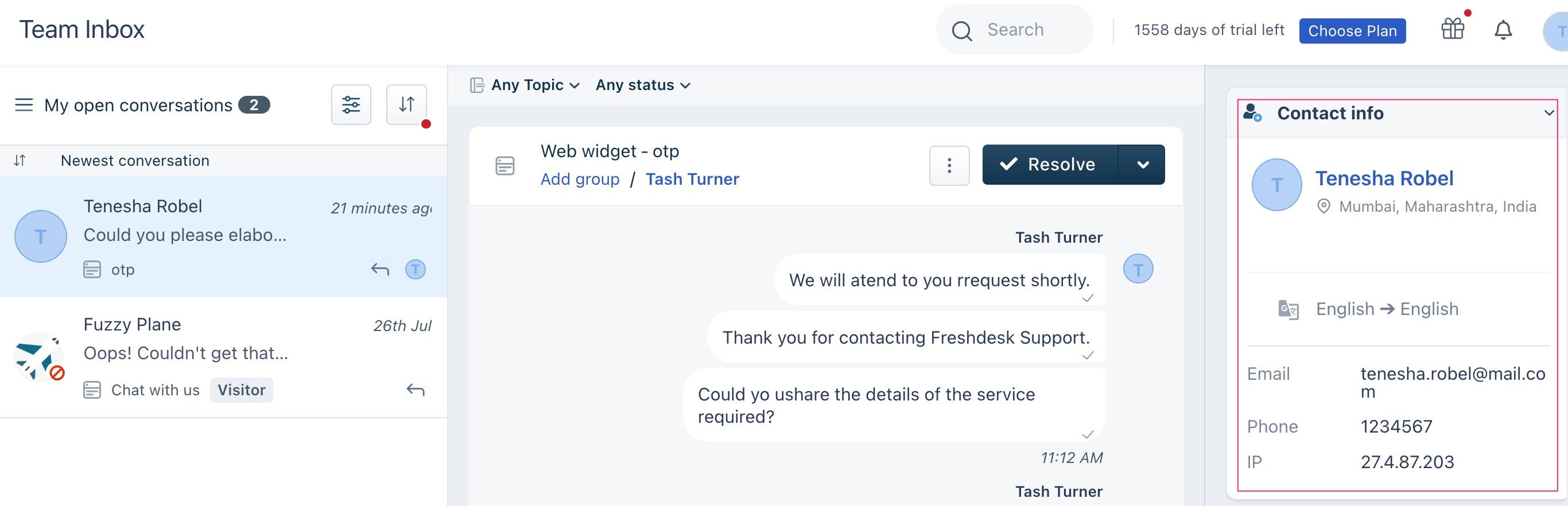
An app can act on the following elements (fields) of the Contact info widget.
Field (default display) name | Element name to be used in app.js | Method name to be used in app.js |
---|---|---|
userEmail | show or hide | |
Phone | userPhoneNumber | show or hide |
IP | userIPAddress | show or hide |
The location information | userLocation | show or hide |
The edit icon (If the app is deployed on a Freshchat account with the <sub-domain>.freshchat.com account URL.) | userEmail, userPhoneNumber, or userIPAddress | enable or disable |
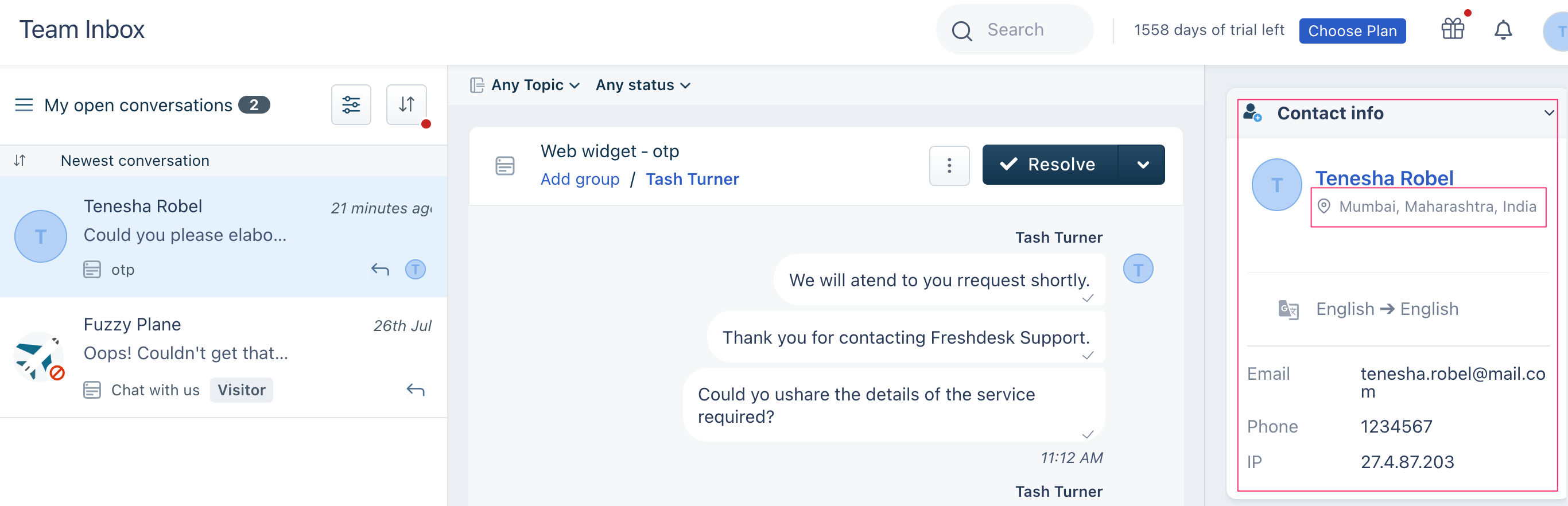
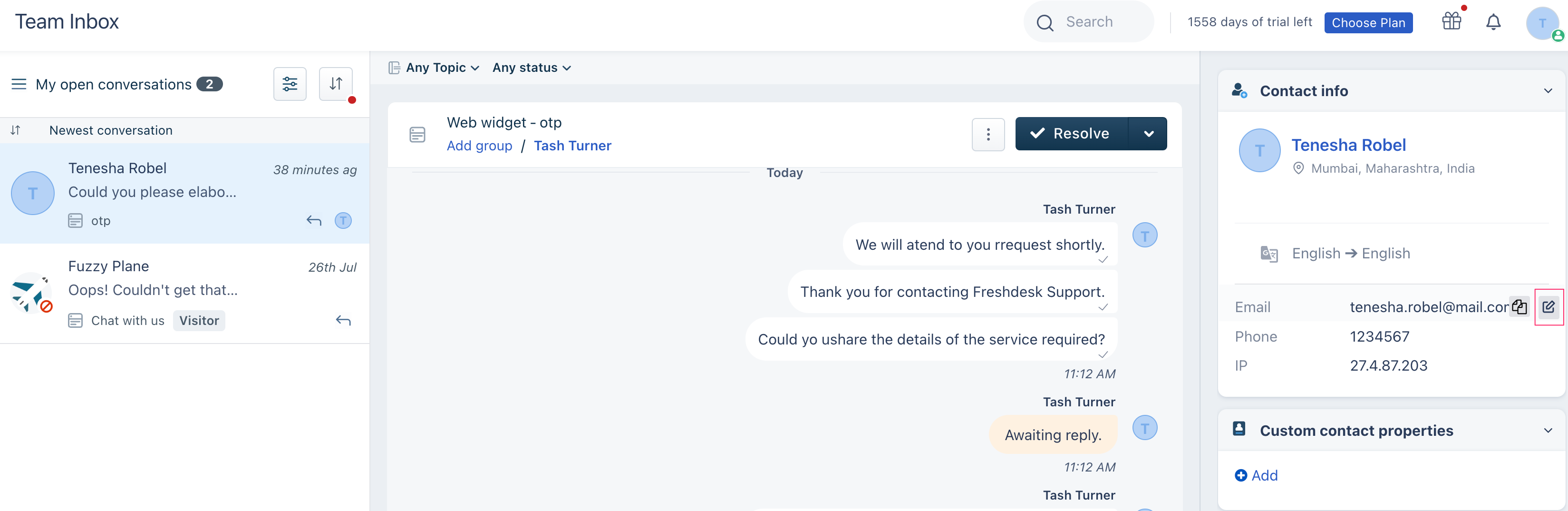
Use the sample code on the right pane, with the appropriate element name, to hide, display, disable, or enable these fields.
Custom contact properties widget
Note:Valid only for apps deployed on the <sub-domain>.freshchat.com account URLs.
The product UI displays all custom information pertaining to the contact (user) associated with a conversation in the Custom contact properties widget of the Team Inbox page. The widget is highlighted in the image.
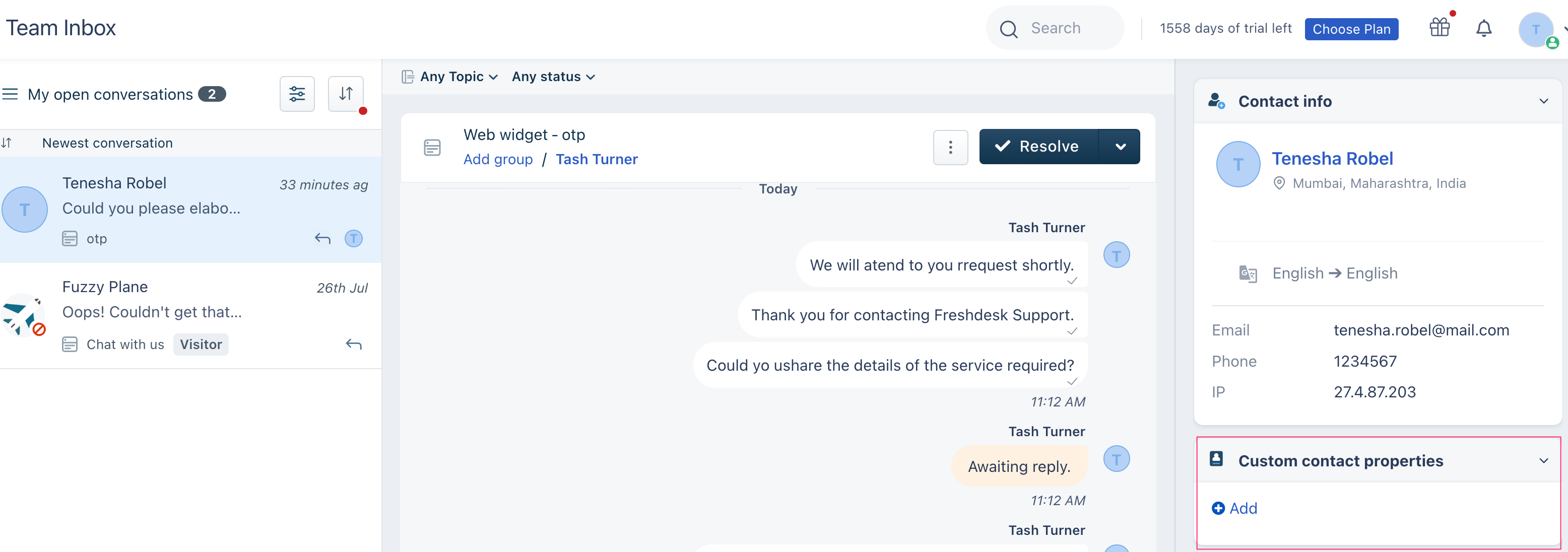
An app can,
- Hide or display the Custom contact properties widget.
- Enable or disable the provision to add more Custom contact properties.
Widget or button (default display) name | Element name to be used in app.js | Method name to be used in app.js |
---|---|---|
Custom contact properties | userCustomProperties | show or hide |
(If the app is deployed on a Freshchat account with the <sub-domain>.freshchat.com account URL.)e | userCustomProperties | enable or disable |
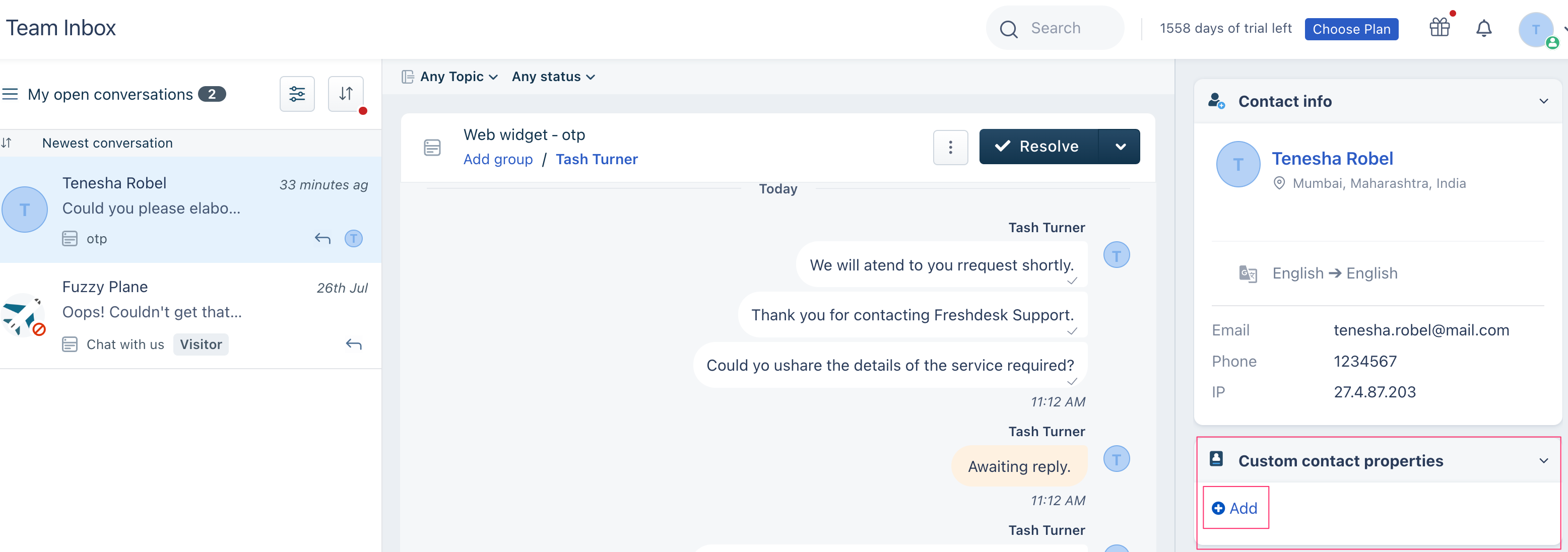
Use the sample code on the right pane, with the appropriate element name and method name, to hide, display, disable, or enable the Custom contact properties widget and the provision to add more Custom contact properties.
Editor window field
When an agent replies or adds a note to a conversation, the editor is displayed.
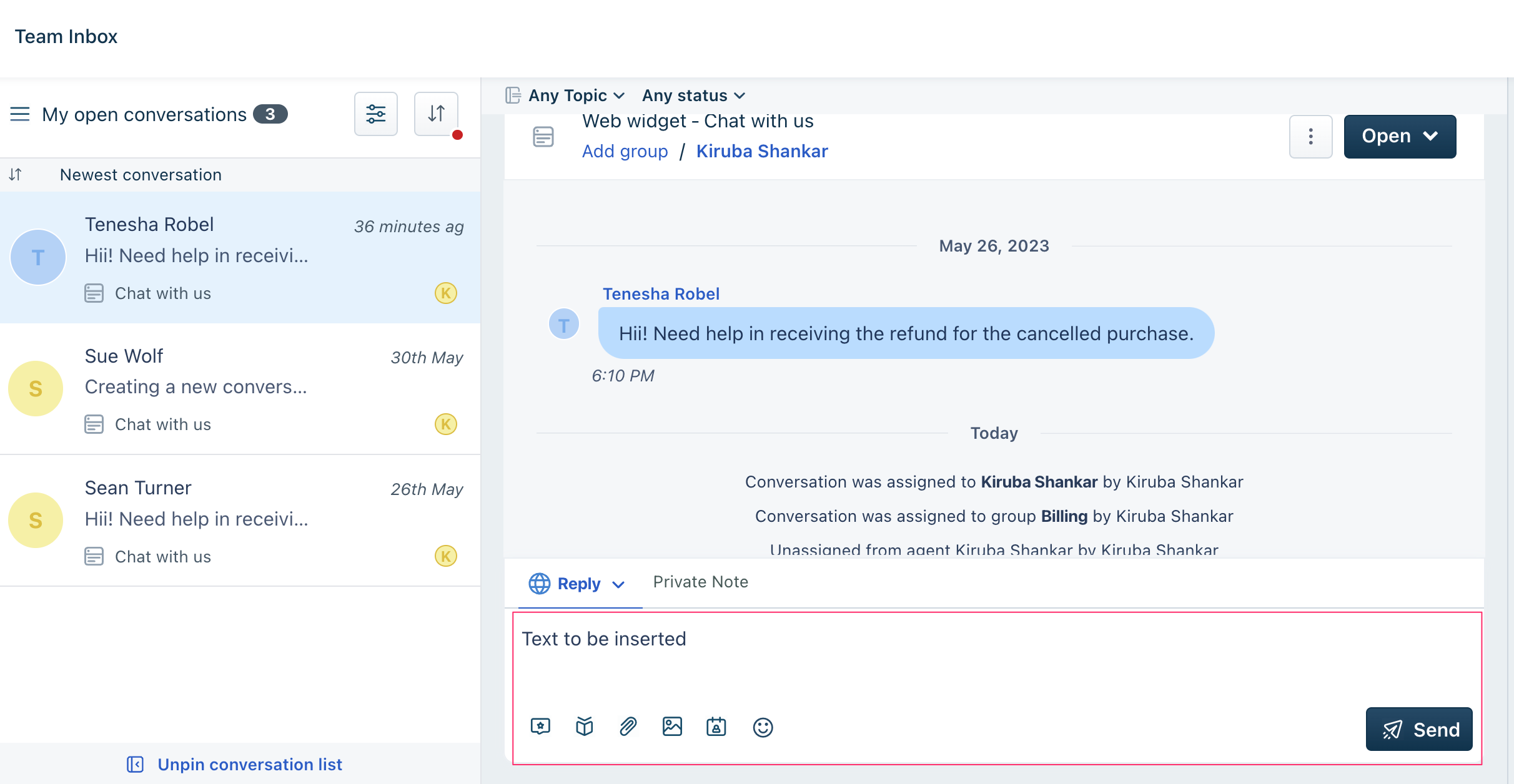
An app can set a text to be displayed on the editor window, on opening. Use the sample code on the right pane to specify a text insert on the Editor window or clear all text inserts.