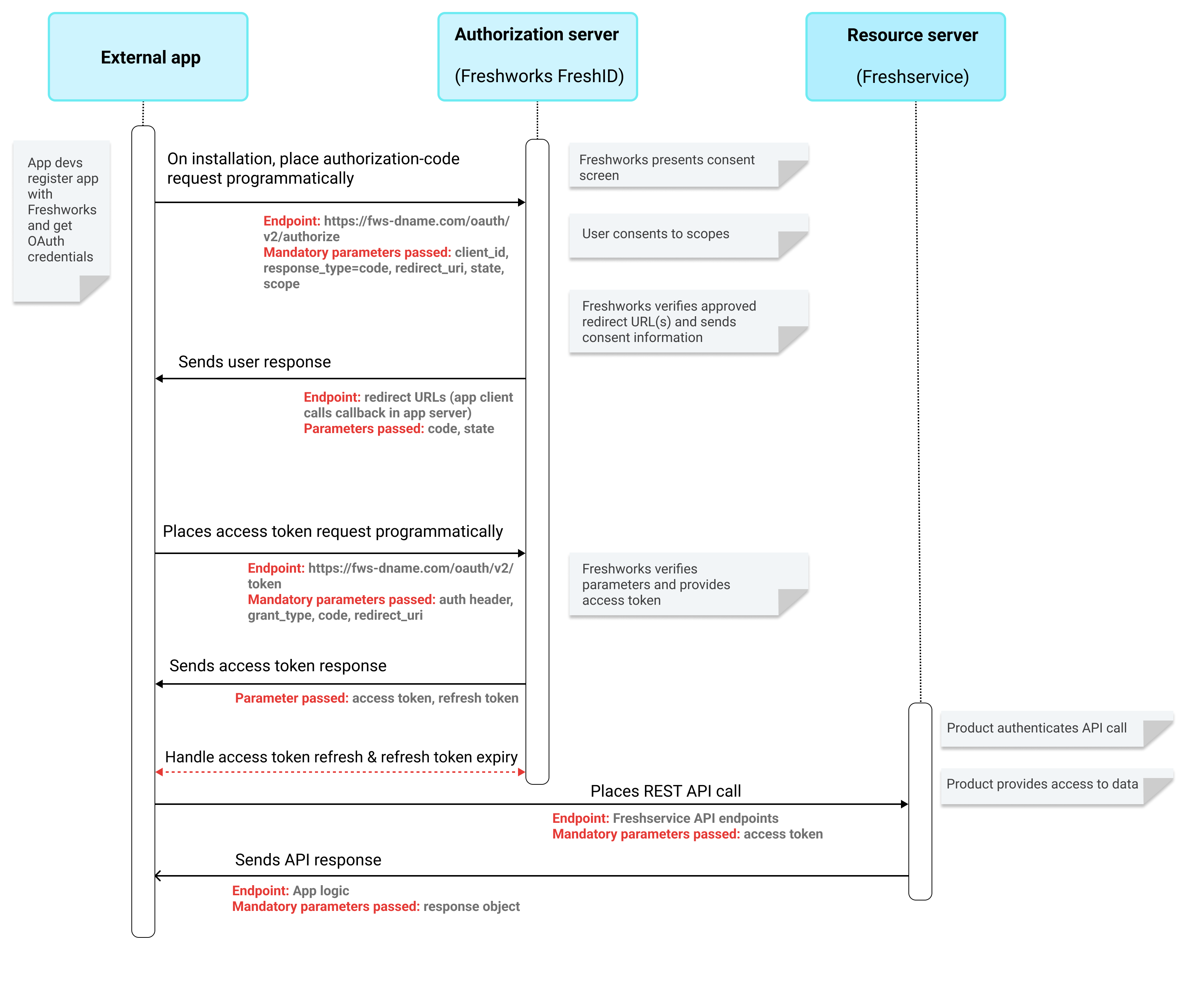
To implement the OAuth flow in your non-Freshworks app that accesses Freshservice resources,
- Create the OAuth credentials for your app.
- Include the requisite code in your app logic to programmatically implement the OAuth flow.
- Test the OAuth flow from your third-party application.
- Publish the app to the third-party Marketplace.
- Submit your app as an External app to the Freshworks Marketplace.
Implement OAuth flow through app code
To do this,
Place authorization-code request: In your app logic, include a UI action such as "Authorize now," which, when clicked, sends app users to the authorization server. The authorization-server URL to which the authorization-code request should be sent: https://fws-dname.com/oauth/v2/authorize.
fws-dname.com is your Freshworks org URL. For information on this, see What is organization URL?
What parameters should be part of the authorization-code request?
Request parameters Description response_type
Mandatory
Type of response that the authorization server should return to the client. Set it as code to denote the authorization code grant type.
client_id
Mandatory
Your app’s unique identifier obtained as part of OAuth credentials creation.
state
Mandatory
Randomly generated alphanumeric string that is used to maintain state between the request and the callback.
scope
Mandatory
Level of access (to the Freshworks resources) that your app requires. You may include all or a subset of the scopes as selected in the OAuth credentials. Ensure that you do not include scopes that are not selected in your OAuth credentials.
For example, for your OAuth credentials, consider that you have selected the following scopes:
- freshservice.solutions.view
- freshservice.solutions.publish
- freshservice.solutions.manage
Therefore, in this attribute, you can specify any or all of the defined scopes, separated by spaces.
Examplescope=freshservice.solutions.view freshservice.solutions.publish freshservice.solutions.manage
redirect_uri
Mandatory
Redirect URL specified when creating OAuth credentials; used for sending the callback.
Sample request URL with parametershttps://fws-dname.com/org/oauth/v2/authorize?response_type=code&state=<random alphanumeric string>&client_id=<your_client_id>&scope=<space separated scopes>&redirect_uri=<redirect_uri>
Sample HTML code for Authorize button<a href="https://fws-dname.com/org/oauth/v2/authorize?response_type=code&state=<random alphanumeric string>&client_id=<your_client_id>&scope=<space separated scopes>&redirect_uri=<redirect_uri>">AUTHORIZE</a>
Receive authorization code: When Freshworks receives a successful authorization-code request call, Freshworks displays the Authorization consent form. The app user response is sent to the redirect URL sent in the authorization-code request.
Sample response call with parameters: <redirect_url>?code=4absj7xxv2h&state=10XXXX
In your app’s client-side code, include the logic to call a callback handler and pass the authorization code received from the Freshworks Authorization server.
Place access-token request: In your app’s server-side code include the callback-handler logic. The handler should handle the response from the authorization server and place the access-token request. The authorization-server URL to which the access-token request should be sent: https://fws-dname.com/oauth/v2/token
fws-dname.com is your Freshworks org URL.
What parameters should be part of the access-token request?
Header parameter Description Authorization
Mandatory
Basic token used for authentication. The format of the token will be the base64 encoding of client_id:client_secret.
ExampleAuthorization: Basic ZnXXXXXXXXXXXXXXXXXXXXXXXXXTQ5N
Request parameters Description code
Mandatory
Authorization code received from Freshworks Authorization server.
redirect_uri
Mandatory
Redirect URL specified in the authorization request.
grant_type
Mandatory
Value: authorization_code
Currently, Freshworks supports only the Authorization-code-grant-type OAuth.
Sample access-token request (in CURL) with request body parameterscurl --location 'http://fws-dname.com/org/oauth/v2/token' \ --header 'Content-Type: application/x-www-form-urlencoded' \ --header 'Authorization: Basic ZndfZXhXXXXXXXXXXXXXXXX==' \ --data-urlencode 'code=WiCXXXXXXXXXXyp_XXXXXXXXVU_SKX4PNUN_DXXXXXXXXWs_07V9-pvXXXXXXXXXXXLkC' \ --data-urlencode 'grant_type=authorization_code' \ --data-urlencode 'redirect_uri=https://www.sample.com'
Receive access token: In your server-side code, include the logic to receive the response of the access-token request.
Sample success response with the requisite access token{ "access_token": "APIKEYVALUEFORAPIV2ACCESS", "refresh_token": "REFRESHTOKENVALUEFORAPIV2ACCESS", "token_type": "Bearer", "scope": "freshservice.solutions.view freshservice.solutions.publish freshservice.solutions.manage", }
Place REST API calls to access Freshservice resources: If the scope your app requested when placing the access-token request call is granted, you can access the Freshservice resources securely through REST API calls by specifying the retrieved access token.
Sample CURL API request that uses the retrieved access tokencurl https://domain.freshservice.com/api/v2/tickets/20' -X GET -H "Content-Type: application/JSON" -H "Authorization: Bearer <retrieved_access_token>"
Handle token lifetime: The access token has a lifetime of 30 minutes. At a regular interval, your app should send a refresh-access-token call to the authorization server and regenerate the access token. When placing a refresh-access-token call, the app should pass the refresh token as a mandatory parameter. The refresh token has a lifetime of 365 days. After the refresh token expires, the access token is not updated and all REST API calls fail with a 401 - Unauthorized error.
To handle this,
- If your app has a front-end component and is installed at a single-user level, in your app code, include the logic to display a Reauthorize button on receiving the 401 error. On-click the app should trigger the authorization code request and regenerate the access and refresh tokens.
- If your app does not have a front-end component, include a logic to trigger the authorization flow for getting a new refresh token.