Interface-methods is a mechanism that the developer platform provides, to enable your app to trigger certain actions on the Freshservice UI.
Using interface methods an app can, on the Freshservice UI,
- Display certain UI elements such as Modals, Confirmation boxes, and Notifications.
- Mimic click actions - such as starting or stopping a timer or navigating to specific pages.
- Show/Hide certain fields, field values, and elements (such as icons or other relevant information).
- Enable/Disable certain buttons.
- Set the values of certain fields.
Global interfaces
An app can display these interfaces/UI elements on any of the Freshservice pages where the app is deployed.
Display modals
From your project’s root, navigate to the app directory and create a <modal or any-reasonable-name>.html file. In this file, enter the code to render the (front-end of the) Modal.
Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
After app initialization, to display a Modal, use the following method:
Sample app.jsclient.interface.trigger("showModal", { title: "<Modal name>", template: "<path to the modal.html file>" })
showModal method
The method displays a Modal in an IFrame. Events methods and Interface methods are not supported in a Modal - your app cannot react to click, change, or update events that occur inside a Modal and your app cannot trigger actions on the product UI from the Modal.
Installation parameters, Request method, Data method, and Data storage are supported in modals. If your app uses these methods from modals, in the modal.html file (or in any other equivalent file that you have added), ensure to include the following appclient resource.
<script src="{{{appclient}}}"></script>
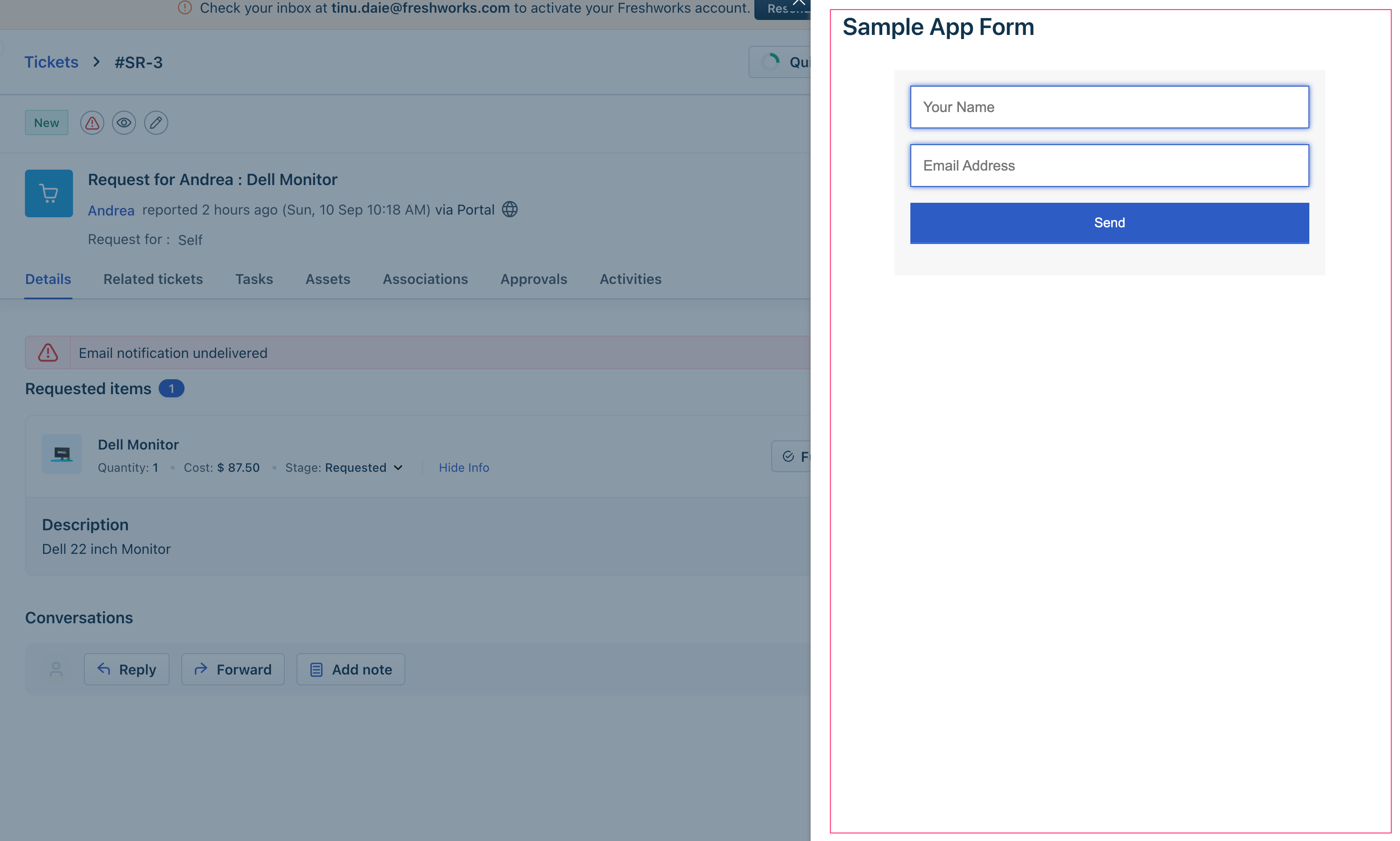
Use the sample code shown on the right pane, to enable your app to display a Modal, as part of the app logic.
You can retrieve the contextual information pertaining to the parent location from where a modal is triggered by using Instance method - Context. You can use the Instance methods - Send and Receive to enable communication between modals and any other app instance.
Display confirmation messages
To enable your app to display standard confirmation-message boxes,
- Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
- After app initialization, to display a confirmation message with default buttons, use the following method:
client.interface.trigger("showConfirm", {
title: "<Confirmation box name>",
message: "<text message to be displayed seeking confirmation>"
})
showConfirm method
The method displays a confirmation message box with a title, a message, and the save and cancel buttons. By default, the message box displays the SAVE and CANCEL buttons. You can customize the button names.
Time-out information:Timeout for Confirmation message box is 10 seconds.
Use the sample code shown on the right pane > Default buttons tab, to enable your app to display a Confirmation message box (with SAVE and CANCEL buttons), as part of the app logic.
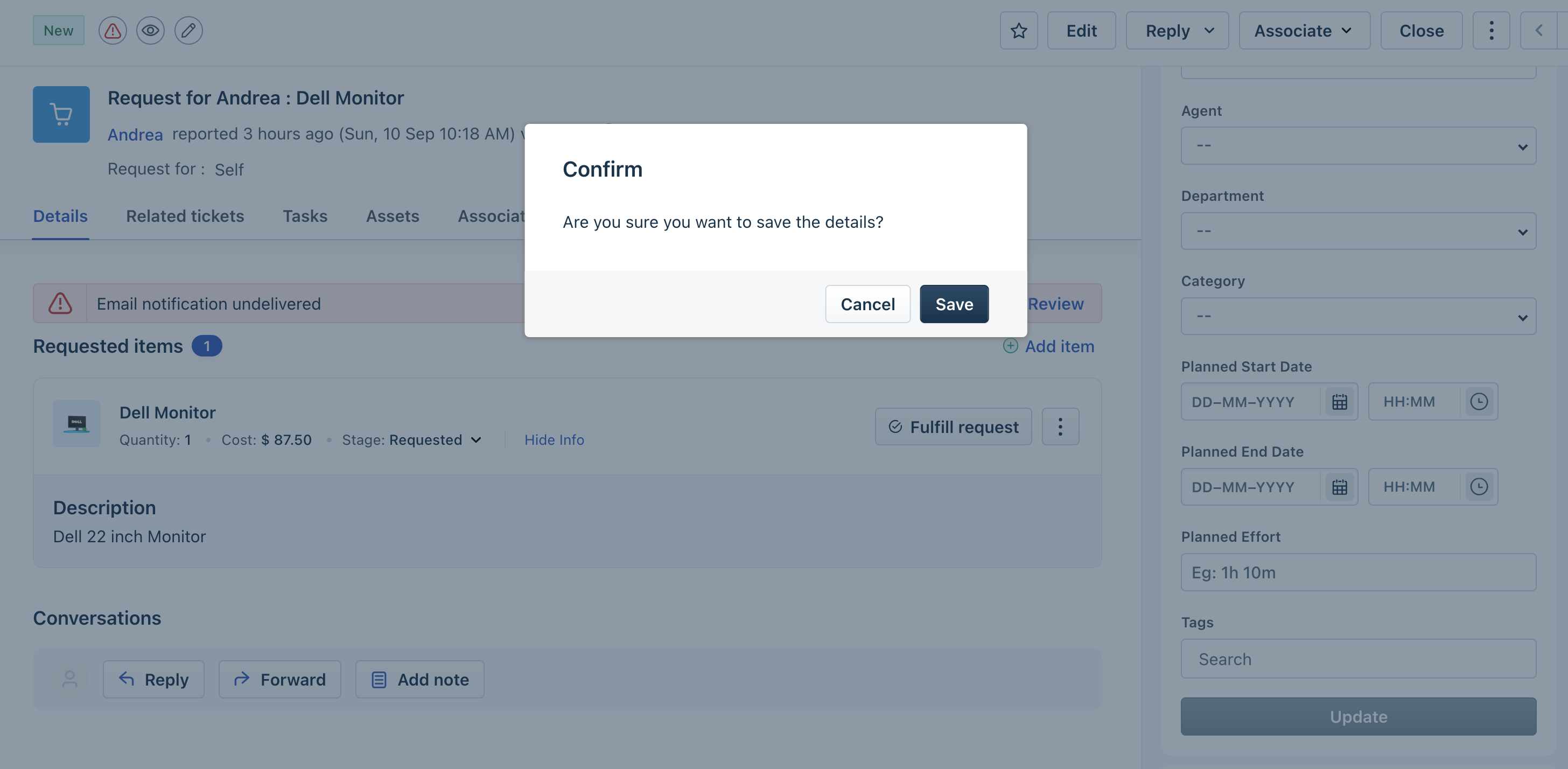
Display notifications
To enable your app to display notifications,
Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
After app initialization, to display a notification, use the following method:
Sample app.jsclient.interface.trigger("showNotify", { type: “<Possible values: info, success, warning, error>”, title: "<Display name>", message: "<text message to be displayed in the notification box>" } )
showNotify method
The method displays a notification box with a title and notification message (can be an info message, a success message, a cautionary note, an error message which, if ignored, can fail the system).
Use the sample code shown on the right pane, to enable your app to display various notification messages, as part of the app logic.
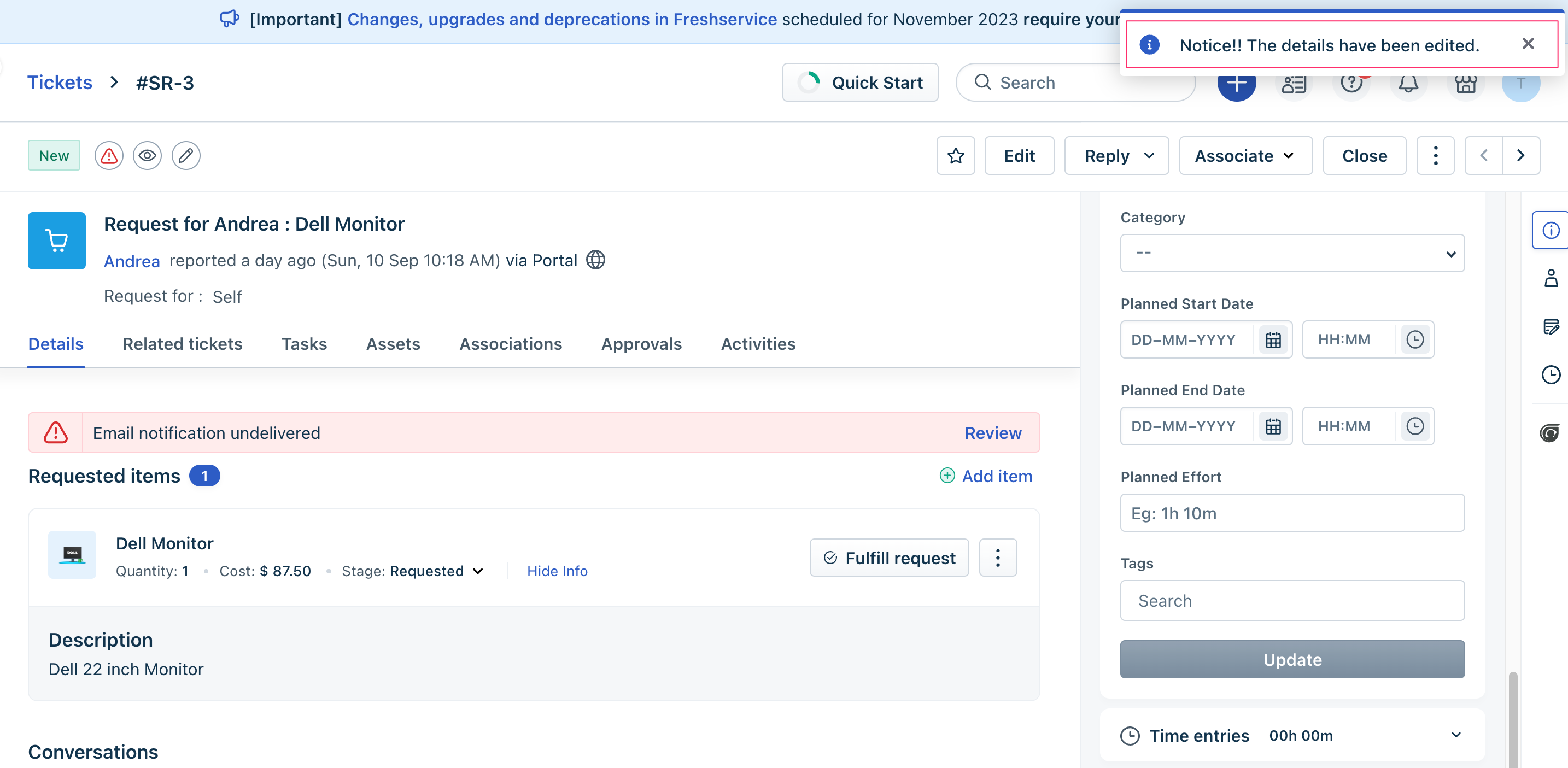
CTI dialler widget- possible app actions
An app deployed at the cti_global_sidebar can display these interfaces on any of the Freshservice pages.
Display or hide CTI dialler
To enable an app deployed at the cti_global_sidebar to open or hide a phone dialler,
- Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (product on which the app is deployed) passes a client reference to the app.
- After app initialization, to open or hide the dialler in an iFrame, use the following method:Sample app.js
client.interface.trigger("<showCtiElement or hideCtiElement>", { id: "softphone" })
show or hide method - for dialler
Use the sample codes shown on the right pane, to enable your CTI app to open or hide the phone dialler in an iFrame.
Display or hide missed calls
To enable an app deployed at the cti_global_sidebar to display or hide the number of missed calls on the CTI widget,
Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (product on which the app is deployed) passes a client reference to the app.
After app initialization, to display or hide the number of missed calls, use the following method:
Sample app.jsShow missed callsHide missed callsclient.interface.trigger("<showCtiElement>", { id: "missedCall", value: <number-of-missed-calls> })
show or hide method - for the number of missed calls on the CTI widget
Use the sample codes shown on the right pane, to enable your CTI app to display or hide the number of missed calls on the CTI widget.
Note:value is the number of missed calls that needs to be displayed on the CTI widget, specified as an integer.
Open ticket details page
To enable your app to open the ticket details pages,
Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (product on which the app is deployed) passes a client reference to the app.
After app initialization, to open the ticket details page, use the following method:
Sample app.jsclient.interface.trigger("openTicket", { id: "<id of the ticket to be opened>" })
openTicket method
The method opens the ticket details page of a specific ticket. Use the sample code shown on the right pane, to enable your app to open the ticket details page.
Open requester details page
To enable your app to open the requester details pages,
Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (product on which the app is deployed) passes a client reference to the app.
After app initialization, to open the requester details page, use the following method:
Sample app.jsclient.interface.trigger("openRequester", { id: "<id of the requester>" })
openRequester method
The method opens the requester details page of a specific requester. Use the sample code shown on the right pane, to enable your app to open the requester details page.
Hide/display or enable/disable UI elements
To enable your app to hide an UI element, display a hidden element, disable an element, or enable an element,
- Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
- After app initialization, to a hide/display or enable/disable UI element, use the following method:<method-name> is the action to be performed. Possible values: hideElement, showElement, enableElement, disableElement
client.interface.trigger("<method-name>", { id: "<element-name>" })
<element-name> is the element to be hidden or displayed; disabled or enabled.
Enable/disable editor window element
To enable your app to disable an element or enable an element, on the reply or forward editor,
- Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
- After app initialization, to a enable/disable an editor window element, use the following method:<method-name> is the action to be performed. Possible values: enableElement, disableElement
client.interface.trigger("<method-name>", { id: "<window-name>", field: "<element-name>" })
<window-name> is the action to be performed. Possible values: reply, forward
<element-name> is the element to be disabled or enabled. Possible value: from
Set the value of a field
To enable your app to set the value of a UI field,
- Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
- After app initialization, use the following method:<element-name> is the element whose value is to be set.
client.interface.trigger("setValue", { id: "<element-name>", value: "<value to be set>" })
Set the value of an editor-window field
To enable your app to set the value of an element, on the reply or forward editor,
Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
After app initialization, use the following method:
client.interface.trigger("setValue", { id: "<window-name>", field: "<element-name>", value: "<value to be set>" })
<window-name> is the editor window name. Possible values: reply, forward
<element-name> is the element (on the reply or forward window) on which the app acts. Possible value: from
Mimic click actions
A button click on the product UI, performs an action. For example, button clicks can start a timer, toggle a timer, expand a collapsed item, open a window, and update field values. Your app can mimic certain button clicks and perform the subsequent actions. To enable your app to do this,
- Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
- After app initialization, to mimic a click action, use the following method:Note: If <action to be performed> is updateProperties, all values of the Properties widget’s fields are obtained as the result of the action.
client.interface.trigger("click", { id: "<action to be performed>" })
- To start a timer, use the following method:
client.interface.trigger("start", { id: "timer", value: {<payload needed for the timer action, specified as an object of key:value pairs}})
- To toggle (if started stop or if stopped start) a timer, use the following method:
client.interface.trigger("toggle", { id: "timer" })
On which UI elements can this method work?
On Ticket Details page:
- timer
- expandConversations
- Reply button: Open the reply editor window with a text insert
- Add Note button: Open the note editor with a text insert
- Tasks > Add new button: Open the add task window with a text insert
On the Ticket details page > Properties widget: Update button: Submit updated ticket properties
UI elements that the app can act on
attachments
Use the sample code on the right pane, to hide attachments or display already hidden attachments.
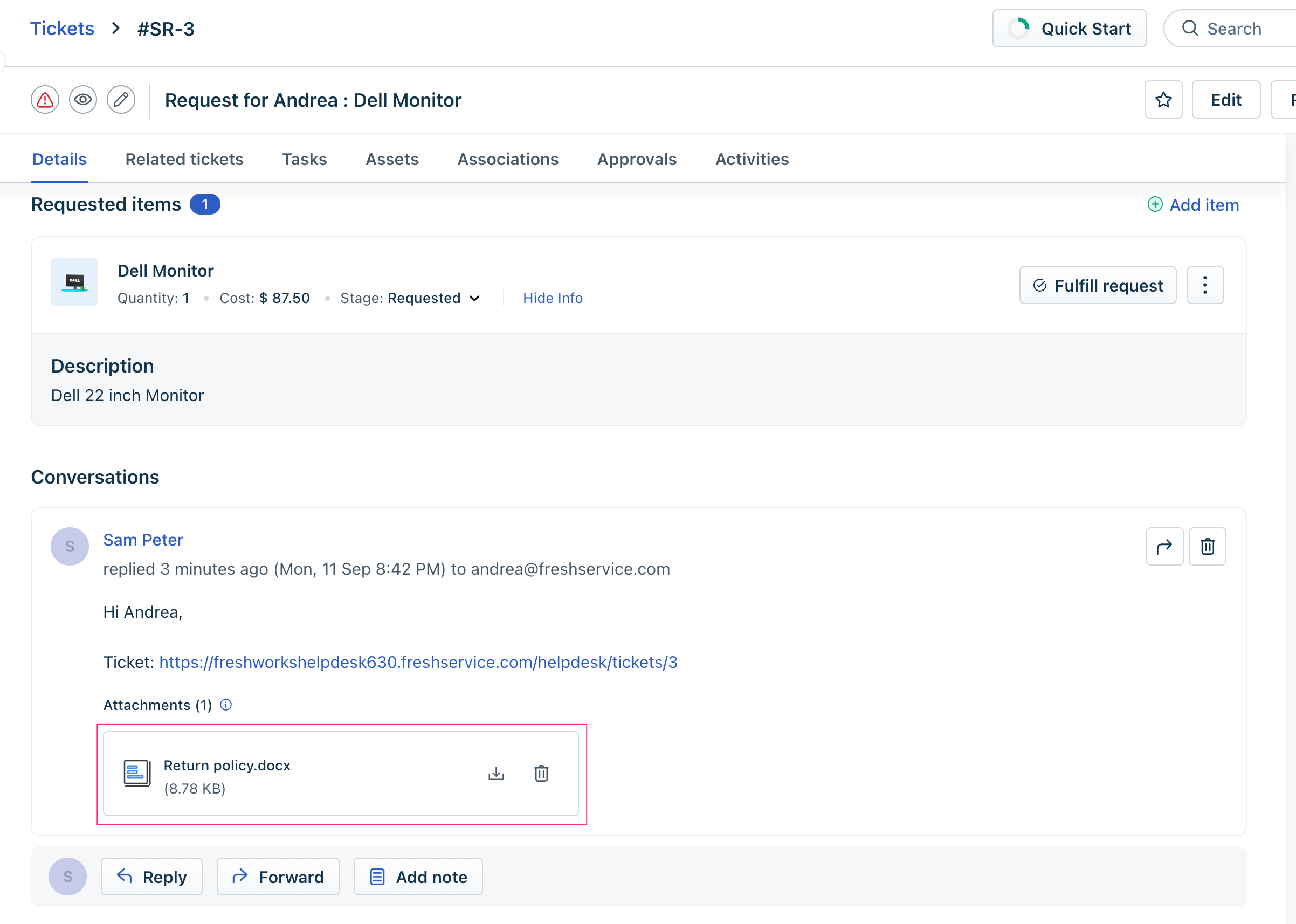
ticketDelete
Use the sample code on the right pane, to hide or display the Delete menu-option from the menu shown in the following image.
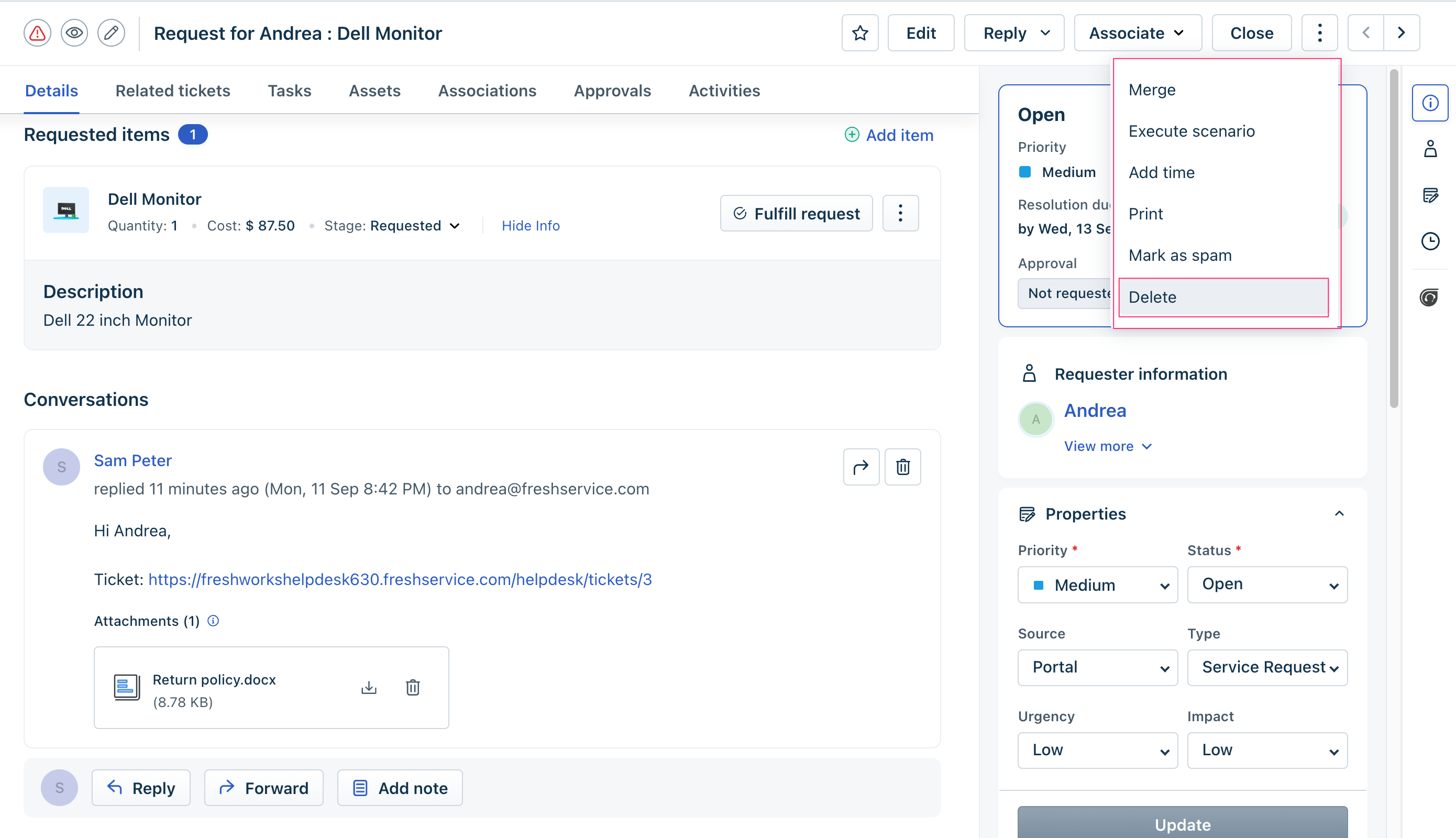
dueDateEdit
The product UI has an edit icon that enables editing the Resolution due date associated with a ticket. The icon is highlighted in the following image. Use the sample code on the right pane, to enable your app to hide or display this link.
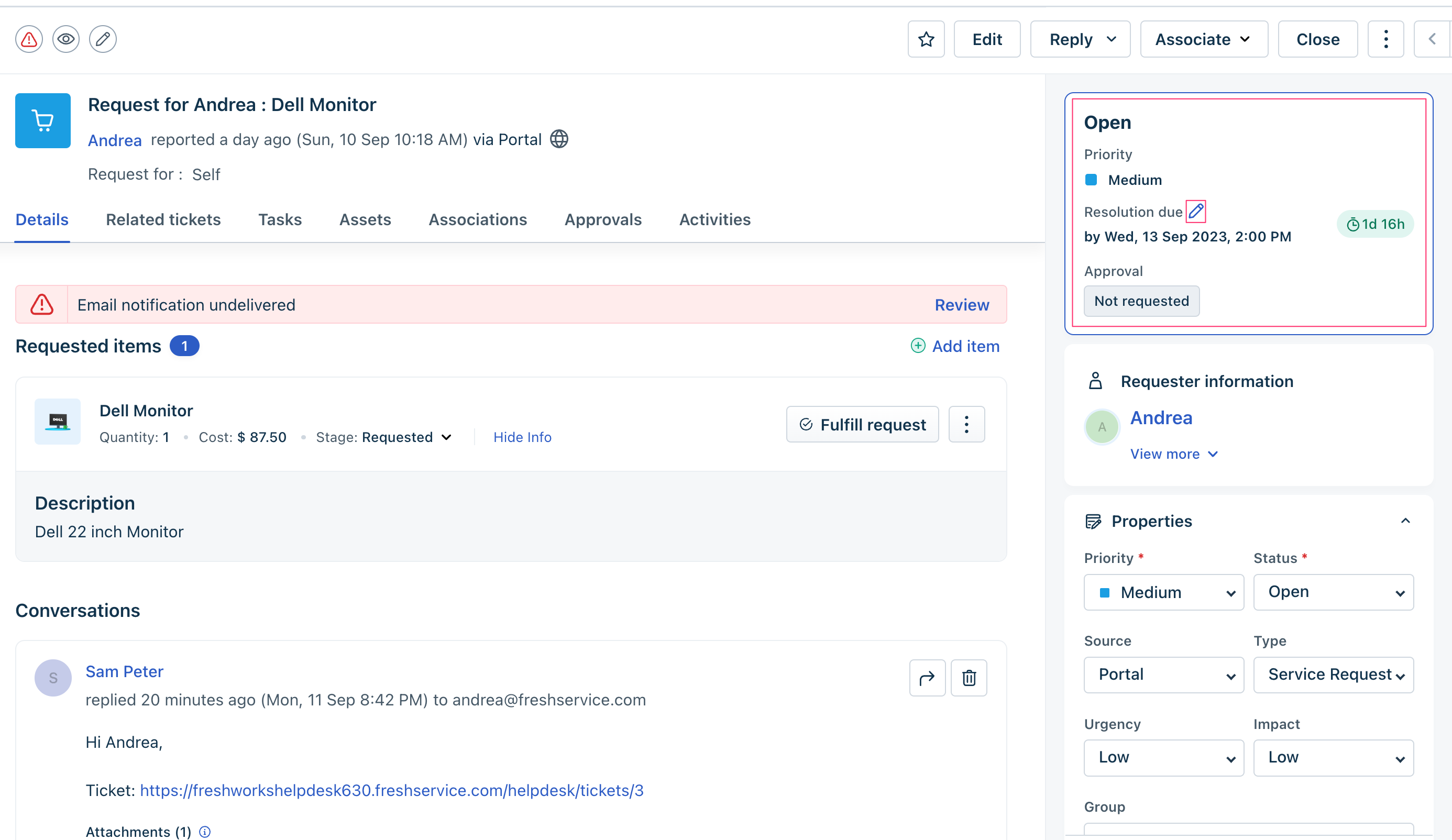
note
Use the sample code on the right pane, to hide/display the Add Note button in the bottom toolbar of the Ticket window and the Add Note drop-down option that is available on clicking the Reply button.
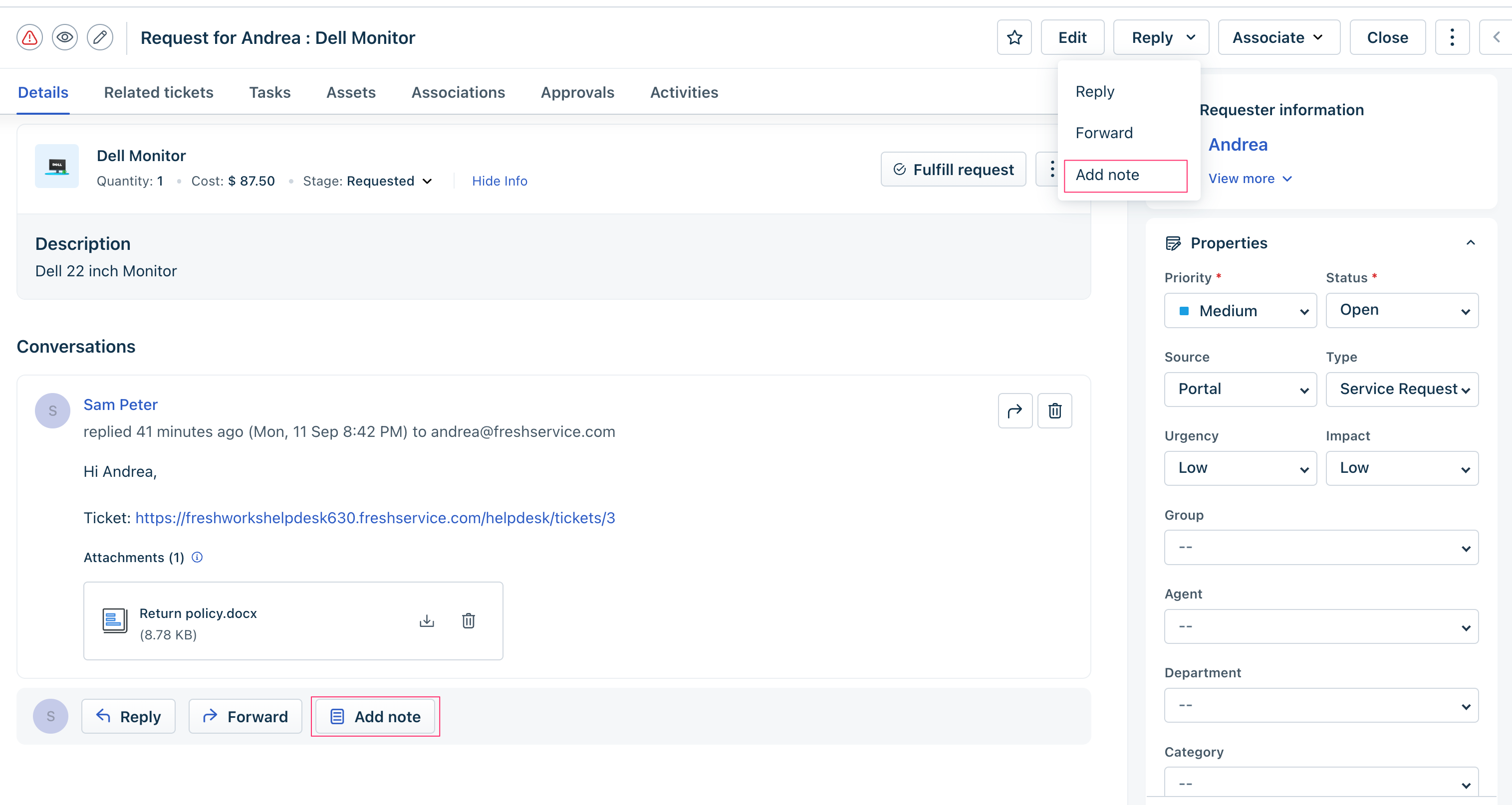
timer
An agent working on a ticket can choose to record the time spent on the ticket. The agent can use the timer available on the product UI, to do this. Your app can mimic the agent’s actions, and start or toggle a timer.
Use the sample code on the right pane, to enable your app to start or stop a timer.
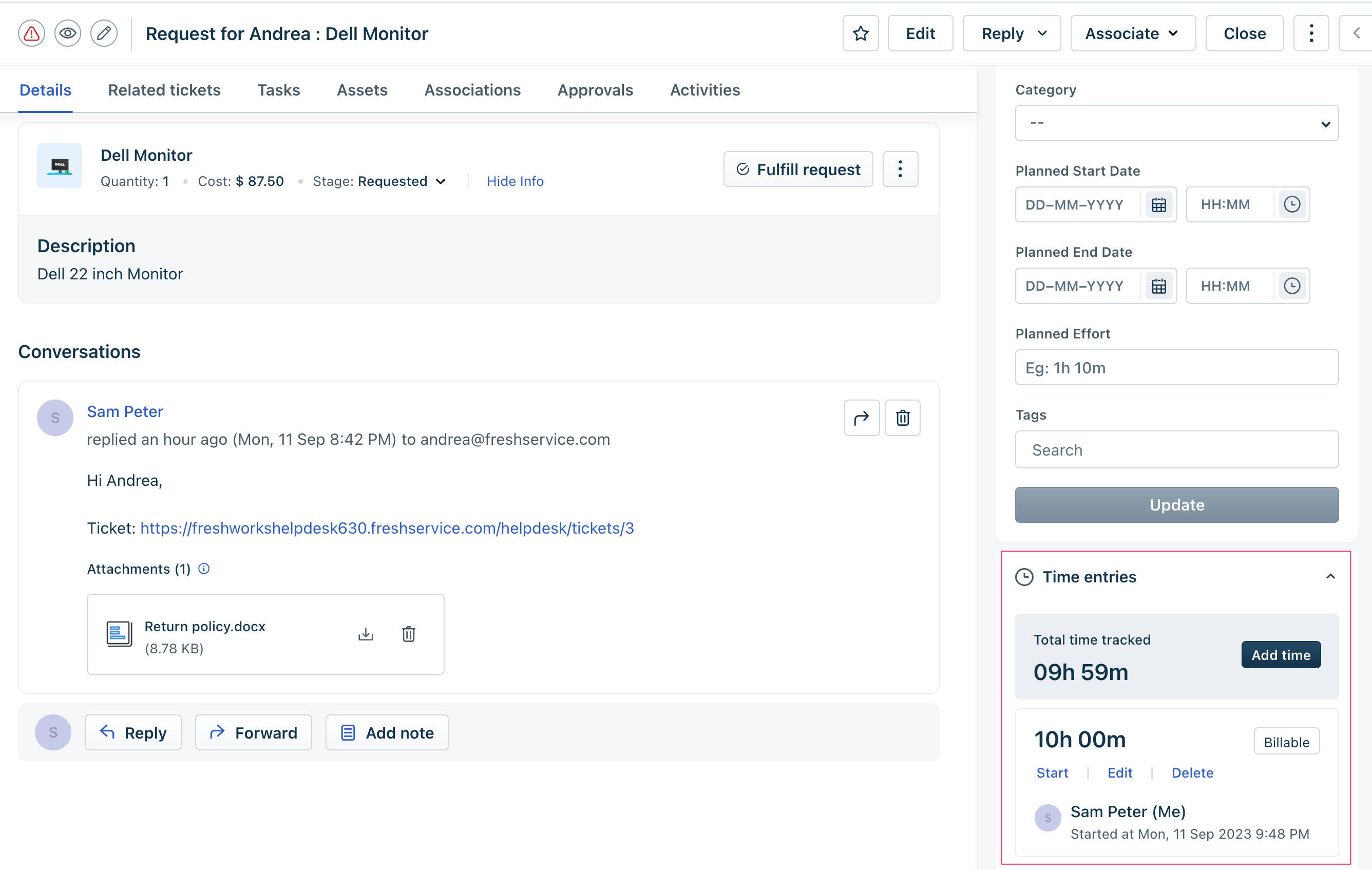
expandConversations
In a ticket thread, if there are more than three messages, they are in collapsed format. Use the sample code on the right pane, to enable your app to expand the collapsed items and let an agent view the entire conversation.
Open the reply editor window with a text insert
Use the sample code on the right pane, to enable your app to mimic a click action on the Reply button and open the reply editor window with a text inserted in the window.
Note:If the ticket.replyClick event method is used in app.js, this interface method triggers the event method and invokes the associated callback.
Open the note editor window with a text insert
Use the sample code on the right pane, to enable your app to open the note editor window with a text inserted in the window.
Note:If the ticket.notesClick event method is used in app.js, this interface method triggers the event method and invokes the associated callback.
Open the add task window with a text insert
Use the sample code on the right pane, to enable your app to open the Add task window with a text inserted in the Note section of the window.
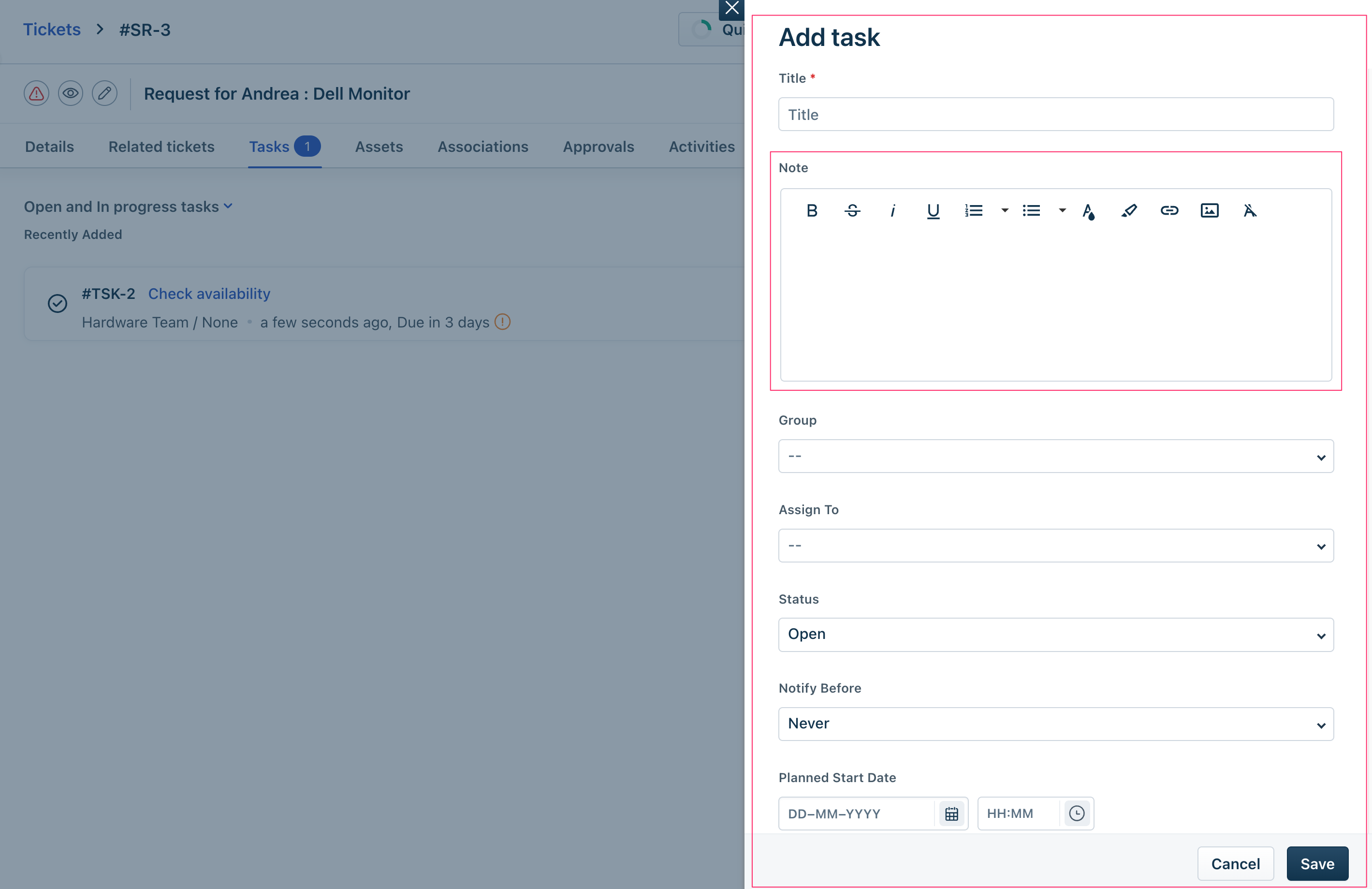
Properties widget fields
The product UI displays all ticket properties in the Properties widget of the Ticket details page. The Properties widget is highlighted in the image.
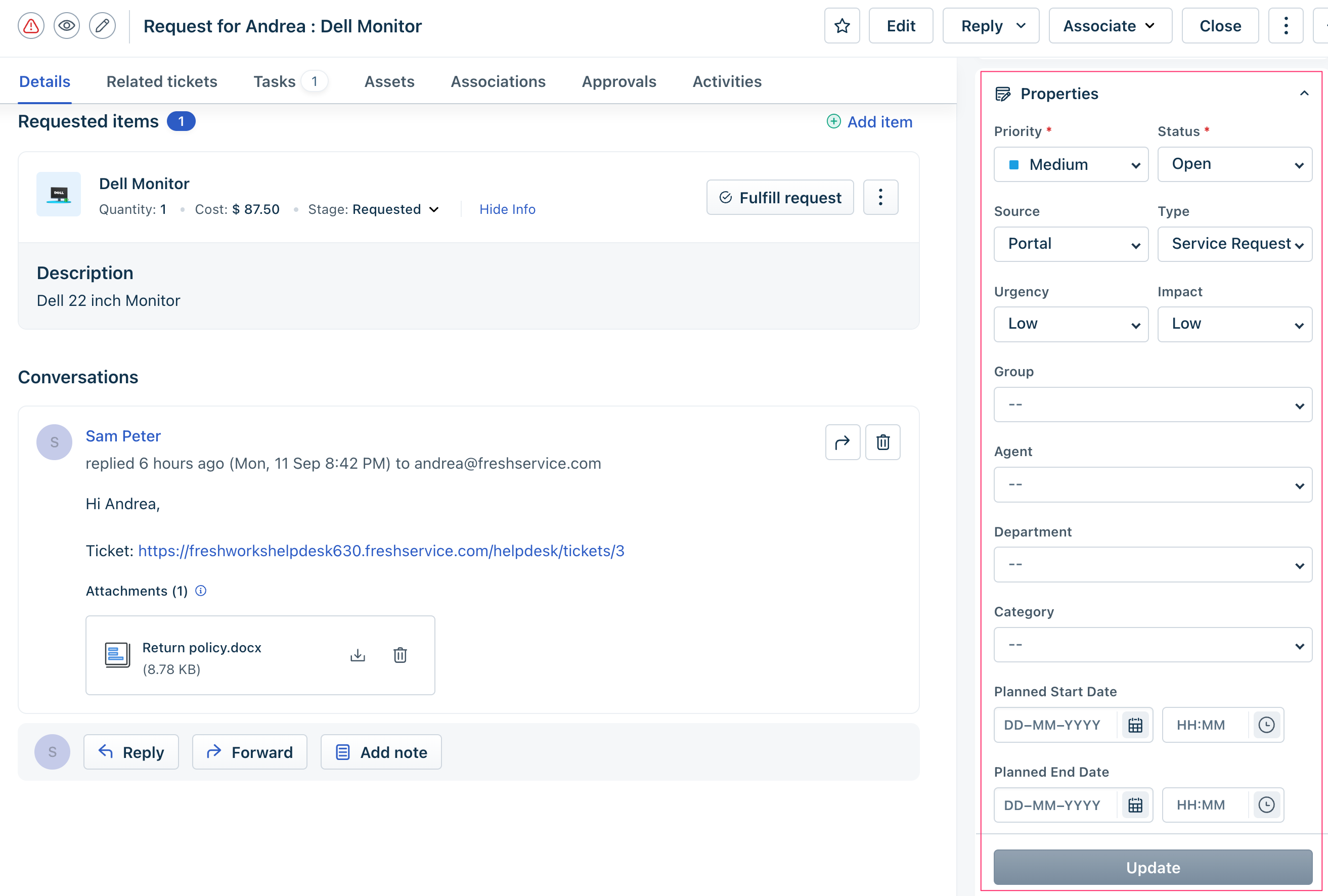
An app can act on the following elements (fields) of the Properties widget.
Field (default display) name | Element name to be used in app.js |
---|---|
Status | status |
Priority | priority |
Source | source |
Impact | impact |
Urgency | urgency |
Type | type |
Agent | agent |
Department | department |
Category | category |
Group | group |
Custom Field | customfield name |
Use the sample code on the right pane, with the appropriate element name, to hide, display, disable, or enable these fields.
setValue: Your app can set a value for a Properties widget field. Use the sample code on the right pane with the appropriate element name, to set a field’s value.
Submit updated ticket properties
You can use the sample code on the right pane to enable your app to mimic a click on the Properties widget > Update button. The values of the properties, at the time of the click, are sent to the app.
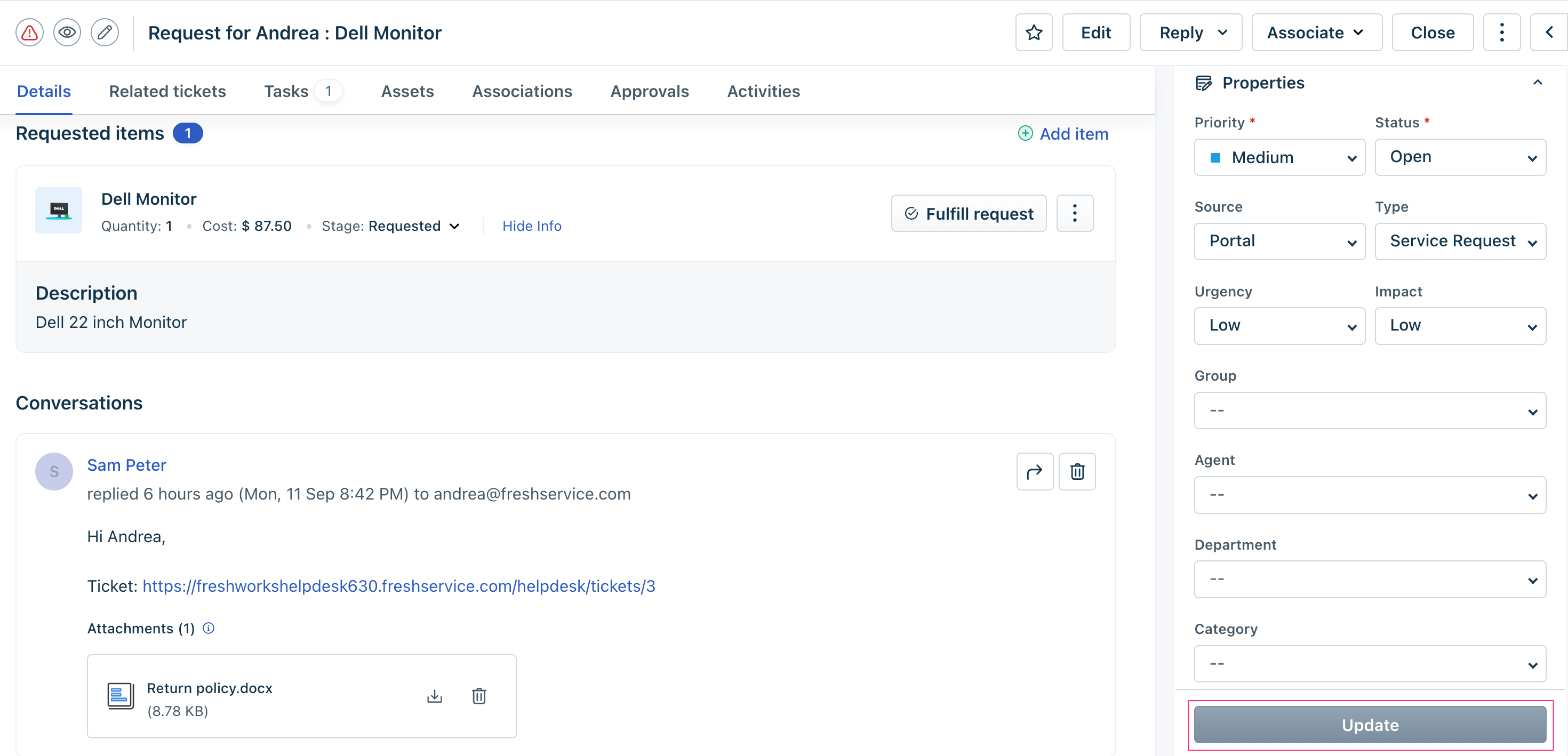
Add note window
When an agent adds a note to a ticket, the Add note editor is displayed. The app can act on the following element of this window.
Use the sample code on the right pane to set the note type. If the note type is private, the Visible to the customer toggle is set to NO.
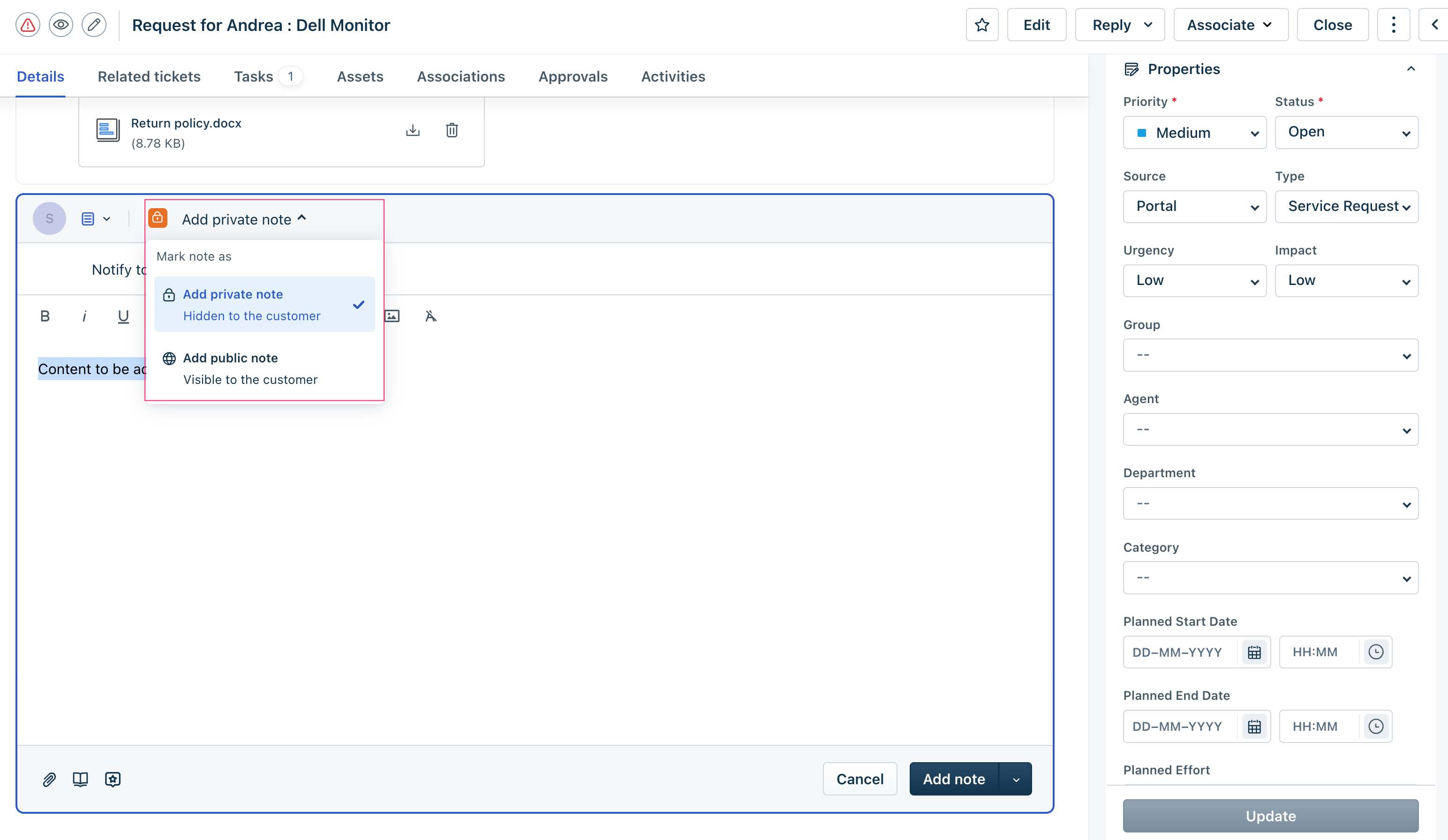
Editor window fields
When an agent replies to or forwards a ticket, the Reply or Forward editor is displayed. The app can act on the following element of the Editor window.
Field (default display) name | Element name to be used in app.js |
---|---|
From | from |
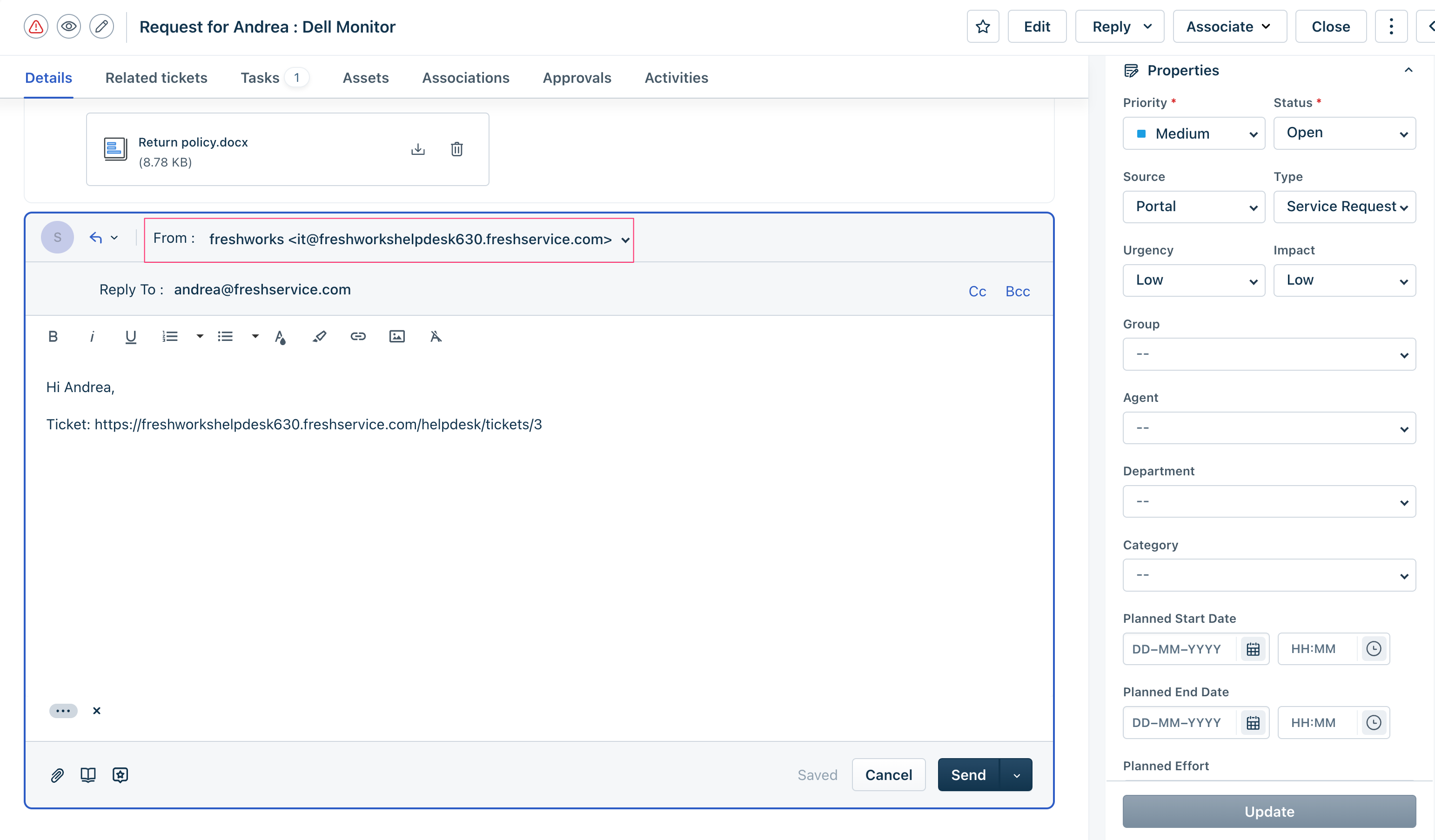
Use the sample code on the right pane, with the appropriate <window-name> to disable or enable the from field.
setValue: Your app can set a value for the Reply or Forward editor’s From field. Use the sample code on the right pane with the appropriate window name, to set the field’s value.
Hide/display UI elements
To enable your app to hide an UI element or display a hidden element,
- Navigate to the app.js file. Subscribe to the app.initialized event, through an event listener. When the app is initialized, the parent application (the product on which the app is deployed) passes a client reference to the app.
- After app initialization, to a hide/display a UI element, use the following method:<method-name> is the action to be performed.
client.interface.trigger("<method-name>", { id: "<element-name>" })
Possible values: hideElement, showElement
<element-name> is the element to be hidden or displayed. Important:To hide/display status, priority, and impact as one unit, enter <element-name> as defaultFields.
UI elements that the app can act on
CHANGE PROPERTIES widget
Any org level service changes can be managed through the Freshservice - Change management. The Change details page captures all information pertaining to a change. The product UI displays all change properties in the CHANGE PROPERTIES widget of the Change details page. The CHANGE PROPERTIES widget is highlighted in the image.
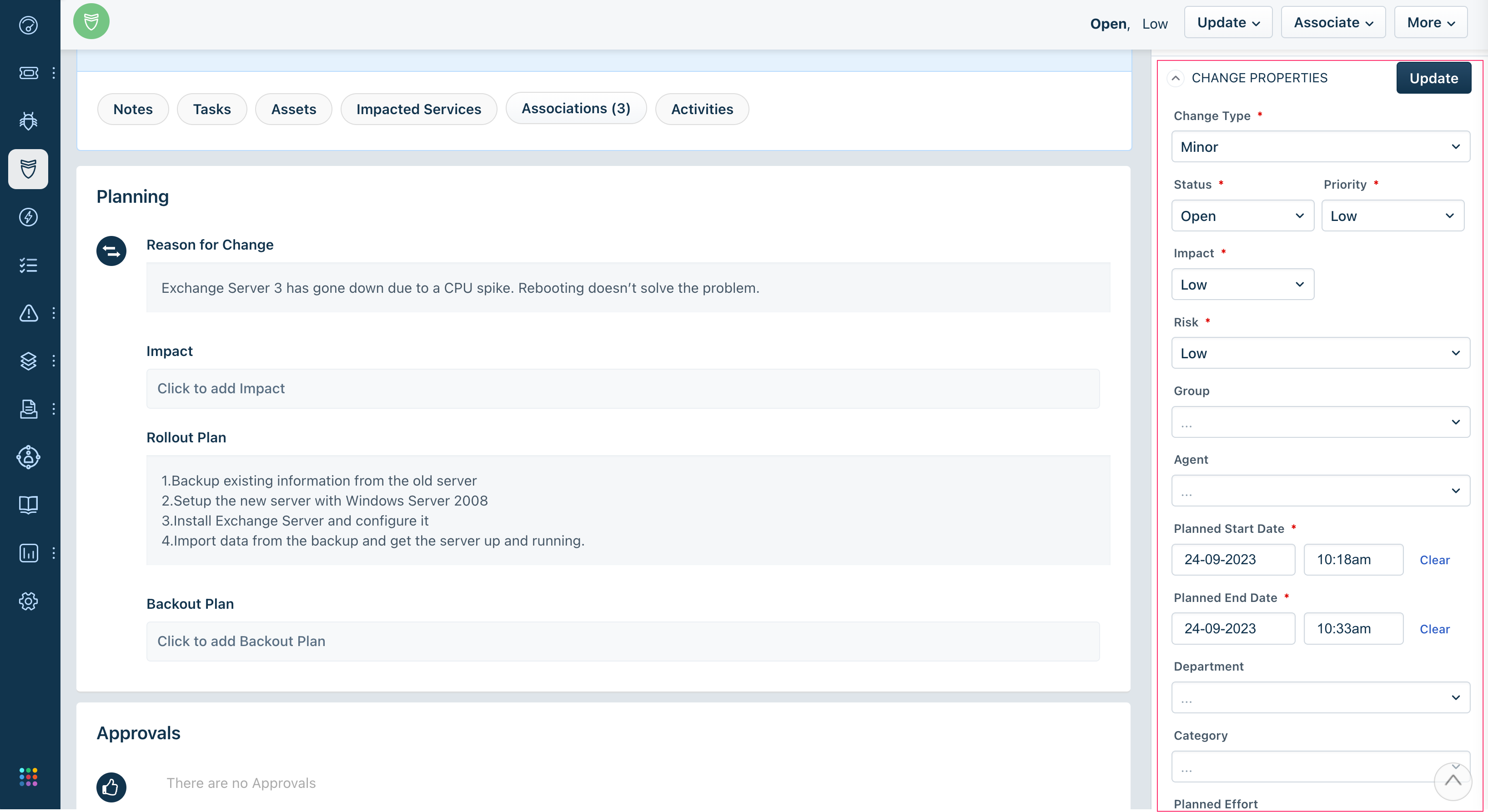
An app can act on the following elements (fields) of the CHANGE PROPERTIES widget.
Field (default display) name | Element name to be used in app.js |
---|---|
Status | status |
Priority | priority |
Impact | impact |
Risk | risk |
Type | type |
Agent | agent |
Department | department |
Category | category |
Group | group |
Planned Start Date | plannedStartDate |
Planned End Date | plannedEndDate |
custom field | customfield |
Use the sample code on the right pane, with the appropriate element name, to hide or display these fields.
setValue: Your app can set a value for a CHANGE PROPERTIES widget field. Use the sample code on the right pane with the appropriate element name, to set a field’s value.
Change view - Planning section
On the Change details page > Change view (that is, the main viewport), the Planning section contains the change roll-out plan. The Planning section is highlighted in the image.
Use the sample code on the right pane, to hide/display or enable/disable (all) Planning section fields
UI elements that the app can act on
The New incident page displays all ticket properties that are necessary for ticket creation.
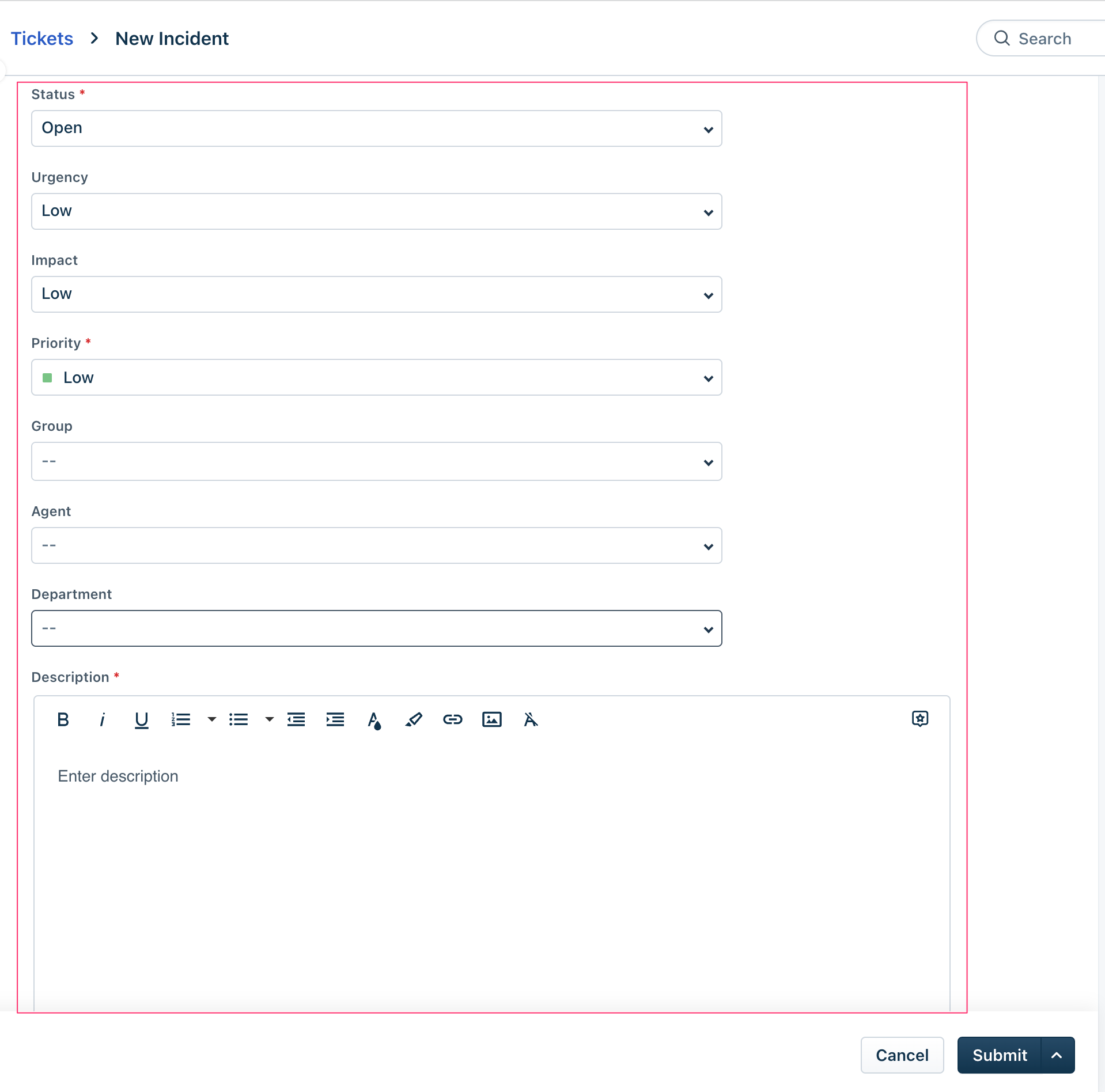
An app can act on the following elements (fields) of the New Incident page.
Field (default display) name | Element name to be used in app.js |
---|---|
Status | status |
Priority | priority |
Impact | impact |
Urgency | urgency |
Agent | agent |
Department | department |
Category | category |
Group | group |
Custom field | customfield |
Use the sample code on the right pane, with the appropriate element name, to hide, display, disable, or enable these fields.
setValue: Your app can set a value for a New Incident page field. Use the sample code on the right pane with the appropriate element name, to set a field’s value.
UI elements that the app can act on
The Create a New Change page displays all change properties that are necessary for a change creation.
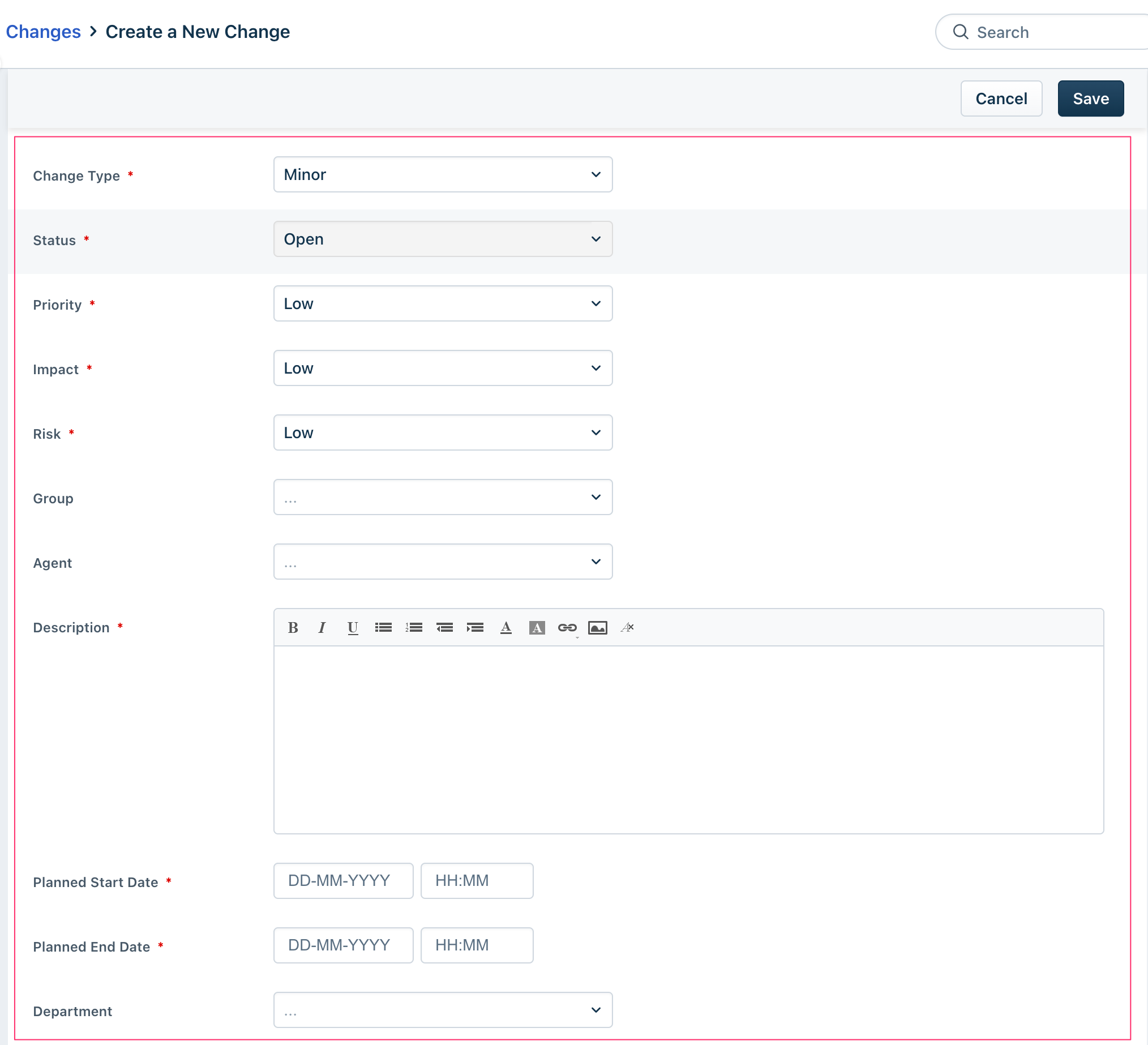
An app can act on the following elements (fields) of the Create a New Change page.
Field (default display) name | Element name to be used in app.js |
---|---|
Change Type | type |
Status | status |
Priority | priority |
Impact | impact |
Risk | risk |
Group | group |
Agent | agent |
Department | department |
Category | category |
Planned Start Date | plannedStartDate |
Planned End Date | plannedEndDate |
Planning (section) | planningFields |
Custom field | customfield |
Use the sample code on the right pane, with the appropriate element name, to hide, display, disable, or enable these fields.
setValue: Your app can set a value for a Create a New Change page field. Use the sample code on the right pane with the appropriate element name, to set a field’s value.